如何使用标准C进行对htm文件中图片的读取与绘图
时间: 2024-01-12 12:02:21 浏览: 21
标准C语言本身并不提供直接读取和绘制HTML文件中图片的功能,但可以通过使用第三方库实现。
对于读取HTML文件中的图片,可以使用第三方库libxml2或者libcurl来解析HTML文件并提取其中的图片信息。
对于绘制图片,可以使用图形库,例如SDL或者OpenGL来实现。
以下是一个使用SDL库读取HTML文件中的图片并显示的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <SDL2/SDL.h>
#include <SDL2/SDL_image.h>
#include <libxml/parser.h>
#include <libxml/xpath.h>
int main(int argc, char *argv[])
{
xmlDocPtr doc;
xmlXPathContextPtr xpath_ctx;
xmlXPathObjectPtr xpath_obj;
xmlNodeSetPtr nodeset;
xmlChar *xpath_expr = (xmlChar*) "//img/@src";
int i;
SDL_Window *win;
SDL_Renderer *ren;
SDL_Texture *tex;
SDL_Surface *bmp;
SDL_Rect rect;
// Initialize SDL
SDL_Init(SDL_INIT_VIDEO);
// Create window and renderer
win = SDL_CreateWindow("Image Viewer",
SDL_WINDOWPOS_UNDEFINED,
SDL_WINDOWPOS_UNDEFINED,
640, 480,
SDL_WINDOW_SHOWN);
ren = SDL_CreateRenderer(win, -1, SDL_RENDERER_ACCELERATED);
// Initialize libxml2
xmlInitParser();
// Parse HTML file
doc = xmlReadFile(argv[1], NULL, 0);
if (doc == NULL) {
printf("Failed to parse document\n");
return 1;
}
// Create XPath context
xpath_ctx = xmlXPathNewContext(doc);
if (xpath_ctx == NULL) {
printf("Failed to create XPath context\n");
return 1;
}
// Evaluate XPath expression
xpath_obj = xmlXPathEvalExpression(xpath_expr, xpath_ctx);
if (xpath_obj == NULL) {
printf("Failed to evaluate XPath expression\n");
return 1;
}
// Get nodeset from XPath object
nodeset = xpath_obj->nodesetval;
// Loop through nodeset and display images
for (i = 0; i < nodeset->nodeNr; i++) {
xmlChar *src = xmlNodeListGetString(doc, nodeset->nodeTab[i]->xmlChildrenNode, 1);
bmp = IMG_Load((char*)src);
if (bmp == NULL) {
printf("Failed to load image %s\n", src);
continue;
}
tex = SDL_CreateTextureFromSurface(ren, bmp);
SDL_FreeSurface(bmp);
xmlFree(src);
if (tex == NULL) {
printf("Failed to create texture\n");
continue;
}
SDL_QueryTexture(tex, NULL, NULL, &rect.w, &rect.h);
rect.x = i * rect.w;
rect.y = 0;
SDL_RenderCopy(ren, tex, NULL, &rect);
SDL_DestroyTexture(tex);
}
// Free XPath object and context
xmlXPathFreeObject(xpath_obj);
xmlXPathFreeContext(xpath_ctx);
// Free document
xmlFreeDoc(doc);
// Cleanup libxml2
xmlCleanupParser();
// Render and wait for user to close window
SDL_RenderPresent(ren);
SDL_Delay(3000);
// Cleanup SDL
SDL_DestroyRenderer(ren);
SDL_DestroyWindow(win);
SDL_Quit();
return 0;
}
```
这段代码使用libxml2库解析HTML文件,提取其中的图片信息,并使用SDL库显示图片。你可以根据自己的需要修改代码,例如将图片保存到文件中,或者使用其他图形库进行绘制。
相关推荐
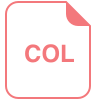
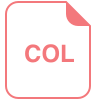
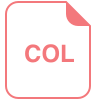
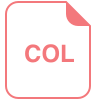
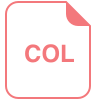









