#include "stdio.h" #include "string.h" int main() { char a[100],b[100],c[100]; printf("please enter No.1:"); fgets(a,100,stdin); printf("please enter No.2:"); fgets(b,100,stdin); printf("please enter No.3:"); fgets(c,100,stdin); char *pa,*pb,*pc,temp; *pa=&a; printf("%s %s %s\n",pa,pb,pc); return 0; }中的*pa=&a为什么会报错
时间: 2024-01-15 09:04:06 浏览: 45
在代码中,对于数组名a,它代表的是数组首元素的地址,是一个指向char类型的指针,因此不需要再加上取地址符&。修改代码如下:
```
#include <stdio.h>
#include <string.h>
int main() {
char a[100], b[100], c[100];
printf("please enter No.1:");
fgets(a, 100, stdin);
printf("please enter No.2:");
fgets(b, 100, stdin);
printf("please enter No.3:");
fgets(c, 100, stdin);
char *pa, *pb, *pc, temp;
pa = a;
pb = b;
pc = c;
printf("%s %s %s\n", pa, pb, pc);
return 0;
}
```
这样就不会报错了。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <windows.h> #include <malloc.h>改成c++
在C++中,`<stdio.h>`、`<stdlib.h>`、`<string.h>`和`<windows.h>`这些头文件在C++标准库中对应的是`iostream`、`cstdlib`、`string`以及`Windows.h`(注意,C++不支持`windows.h`,因为它主要用于Windows API)。为了将这段C代码转换为C++,我们需要做以下调整:
1. 使用`#include <iostream>`替换`#include <stdio.h>`,因为`iostream`包含了输入输出流和基本I/O操作。
2. 使用`#include <cstdlib>`替换`#include <stdlib.h>`,因为`cstdlib`包含了内存管理函数。
3. 使用`#include <string>`替换`#include <string.h>`,因为`string`库提供了字符串处理功能。
4. 删除`#include <windows.h>`,因为C++不支持Windows API,如果需要Windows特定的头文件,需要使用C++的命名空间或头文件,但这里假设你不需要Windows API。
下面是转换后的C++代码:
```cpp
#include <iostream>
#include <cstdlib>
#include <string>
// 使用std::string代替char数组
std::string strchr(const std::string& s, char c) {
auto it = std::find(s.begin(), s.end(), c);
if (it != s.end()) {
return std::string{s.begin(), it};
}
return "";
}
// 使用std::string和strcpy的等价函数std::copy
std::string mystr(std::string& dst, const std::string& str) {
std::copy(str.begin(), str.end(), dst.begin());
dst.resize(dst.size()); // 添加终止符'\0'
return dst;
}
int main() {
std::string s1 = "abc";
std::string s2 = mystr(s1);
std::cout << s2 << std::endl;
return 0;
}
```
#include <unistd.h> #include <string.h> #include <stdio.h> #include <sys/stat.h> #include <fcntl.h>
`#include <unistd.h>`是一个C语言的头文件,它包含了一些与系统调用相关的函数和常量。其中一些常用的函数包括`fork()`、`exec()`和`pipe()`等。`<string.h>`是另一个C语言的头文件,它包含了一些与字符串操作相关的函数,例如`strcpy()`和`strlen()`等。`<stdio.h>`是标准输入输出的头文件,它包含了一些与输入输出相关的函数,例如`printf()`和`scanf()`等。`<sys/stat.h>`是一个系统状态的头文件,它包含了一些与文件状态相关的函数和常量,例如`stat()`和`chmod()`等。`<fcntl.h>`是文件控制的头文件,它包含了一些与文件操作相关的函数和常量,例如`open()`和`close()`等。
以下是一个简单的示例代码,演示了如何使用这些头文件中的函数:
```c
#include <unistd.h>
#include <string.h>
#include <stdio.h>
#include <sys/stat.h>
#include <fcntl.h>
int main() {
// 使用unistd.h中的函数
int pid = fork();
if (pid == 0) {
// 子进程
printf("This is child process.\n");
} else if (pid > 0) {
// 父进程
printf("This is parent process.\n");
} else {
// 出错
perror("fork");
return 1;
}
// 使用string.h中的函数
char str1[10] = "Hello";
char str2[10];
strcpy(str2, str1);
printf("Copied string: %s\n", str2);
// 使用stdio.h中的函数
int num;
printf("Enter a number: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
// 使用sys/stat.h和fcntl.h中的函数
int fd = open("file.txt", O_RDONLY);
struct stat fileStat;
fstat(fd, &fileStat);
printf("File size: %ld bytes\n", fileStat.st_size);
close(fd);
return 0;
}
```
这段代码演示了如何使用`fork()`函数创建子进程,使用`strcpy()`函数复制字符串,使用`scanf()`函数读取用户输入的数字,以及使用`open()`函数打开文件并使用`fstat()`函数获取文件大小。
阅读全文
相关推荐
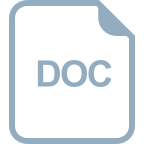
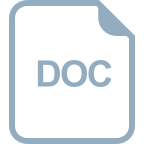
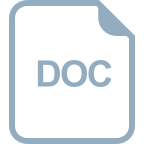
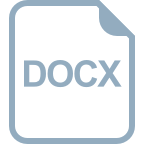













