C++输入一串字符,将其中的大写变成小写,若不为大写则原样输出。
时间: 2023-09-25 15:06:08 浏览: 59
```c
#include <stdio.h>
int main() {
char str[100];
printf("请输入一串字符:");
scanf("%s", str);
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] += 32;
}
}
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
相关问题
输入一串字符,将其中的大写变成小写,若不为大写则原样输出。c++
这里给出一个C语言的实现:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
printf("请输入一串字符:");
gets(str); // 使用 gets 函数输入字符串(不安全,不建议使用)
for(int i=0; str[i]!='\0'; i++) {
if(isupper(str[i])) { // 如果是大写字母
str[i] = tolower(str[i]); // 转换为小写
}
}
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
需要注意的是,这里使用了 `gets` 函数来输入字符串。这个函数并不安全,因为它无法限制输入的字符串长度,可能会导致缓冲区溢出等问题。在实际开发中,应该使用更加安全的函数,如 `fgets` 等。
C加加实现输入一串字符大写转换成小写小写转换成大写其他字符原样输出
```c++
#include <iostream>
using namespace std;
int main() {
char c;
while(cin >> c) {
if(c >= 'a' && c <= 'z') {
c = c - 'a' + 'A';
} else if(c >= 'A' && c <= 'Z') {
c = c - 'A' + 'a';
}
cout << c;
}
return 0;
}
```
说明:
1. 通过 `cin` 读取字符,如果读取到文件结尾或遇到输入错误将退出循环。
2. 判断字符的大小写,如果是小写字母将其转换为大写字母,如果是大写字母将其转换为小写字母。
3. 输出转换后的字符。
相关推荐
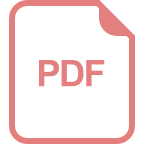
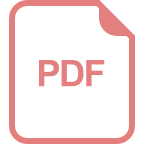
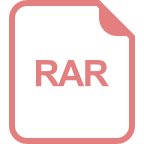











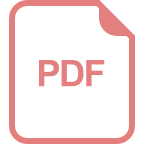