import os import pandas as pd import glob import pandas as pd import numpy as np import matplotlib.pyplot as plt import seaborn as sns %matplotlib inline plt.rcParams['font.sans-serif']=['SimHei'] plt.rcParams['axes.unicode_minus']=Falsecsv_list = glob.glob('all.csv') print(u'共发现%s个CSV文件'% len(csv_list)) print(u'正在处理............') for i in csv_list: fr = open(i,'rb').read() with open('result.csv','ab') as f: f.write(fr) print('合并完毕!')
时间: 2023-09-03 10:08:45 浏览: 70
这段代码的功能是将当前目录下所有后缀名为csv的文件合并成一个名为result.csv的文件。具体来说,首先使用glob.glob('all.csv')获取当前目录下所有后缀名为csv的文件,并将其保存到csv_list列表中。然后使用for循环遍历csv_list列表中的所有csv文件,将其内容读取出来,再写入到名为result.csv的文件中。最后输出“合并完毕!”提示信息。
相关问题
import pandas as pd import matplotlib.pyplot as plt %matplotlib inline import numpy as np
import pandas as pd是Python中用于数据分析和处理的库,它提供了丰富的数据结构和数据分析工具。通过使用pd别名,我们可以方便地引用pandas库中的函数和类。
matplotlib.pyplot是Python中用于绘制图表和可视化数据的库。通过使用plt别名,我们可以方便地引用matplotlib.pyplot库中的函数和类。
%matplotlib inline是Jupyter Notebook中的魔术命令,它用于在Notebook中显示matplotlib绘制的图表。这个命令告诉Notebook将图表直接嵌入到输出单元格中,而不是弹出一个新窗口显示图表。
import numpy as np是导入NumPy库,NumPy是Python中用于科学计算的库,它提供了高性能的多维数组对象和各种数学函数。
import pandas as pdimport numpy as npimport matplotlib.pyplot as pltimport seaborn as sns
这是一段Python代码,它导入了四个库:pandas、numpy、matplotlib.pyplot和seaborn。这些库都是用于数据分析和可视化的常用库。其中,pandas用于数据处理和分析,numpy用于科学计算,matplotlib.pyplot用于绘制图表,seaborn用于数据可视化。如果你想使用这些库,需要先安装它们。你可以使用pip命令来安装它们,例如:
```shell
pip install pandas numpy matplotlib seaborn
```
安装完成后,你就可以在Python代码中使用它们了。
相关推荐
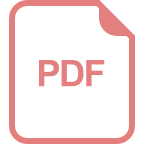
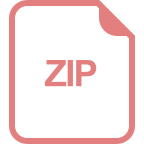













