import cv2 import numpy as np img = cv2.imread('2.jpg') gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 100, param1=100, param2=30, minRadius=5, maxRadius=300) circle = circles[0][0] center = (int(circle[0]), int(circle[1])) radius = int(circle[2]) angle = np.pi / 4 # 45度 point_on_circle = (int(center[0] + radius * np.cos(angle)), int(center[1] + radius * np.sin(angle))) print('圆心坐标:',center) print('圆上一点坐标',int(center[0] + radius * np.cos(angle)) ,int(center[1] + radius * np.sin(angle))) cv2.circle(img, center, radius, (0, 255, 0), 2) cv2.circle(img, center, 2, (0, 0, 255), 3) cv2.circle(img, point_on_circle, 2, (0, 0, 255), 3) cv2.imshow('image', img) cv2.waitKey(0) cv2.destroyAllWindows()请修改上述代码。可以在qt界面中显示
时间: 2023-07-15 14:12:13 浏览: 61
您可以使用以下代码将上述代码转换为在Qt界面中显示的代码:
```python
import cv2
import numpy as np
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QVBoxLayout
class MainWindow(QWidget):
def __init__(self):
super().__init__()
# 读取图像
img = cv2.imread('2.jpg')
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测圆
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 100, param1=100, param2=30, minRadius=5, maxRadius=300)
# 取第一个圆
circle = circles[0][0]
# 获取圆心坐标、半径
center = (int(circle[0]), int(circle[1]))
radius = int(circle[2])
# 计算圆上一点坐标
angle = np.pi / 4 # 45度
point_on_circle = (int(center[0] + radius * np.cos(angle)), int(center[1] + radius * np.sin(angle)))
# 在图像中绘制圆和点
cv2.circle(img, center, radius, (0, 255, 0), 2)
cv2.circle(img, center, 2, (0, 0, 255), 3)
cv2.circle(img, point_on_circle, 2, (0, 0, 255), 3)
# 将图像转换为QImage
img_rgb = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
h, w, ch = img_rgb.shape
bytes_per_line = ch * w
qimg = QImage(img_rgb.data, w, h, bytes_per_line, QImage.Format_RGB888)
# 在Qt界面中显示图像
label = QLabel(self)
pixmap = QPixmap.fromImage(qimg)
label.setPixmap(pixmap)
# 设置窗口布局
layout = QVBoxLayout()
layout.addWidget(label)
self.setLayout(layout)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
这段代码使用了PyQt5库来创建一个Qt界面,并在其中显示图像。您需要安装PyQt5库才能运行此代码。
相关推荐
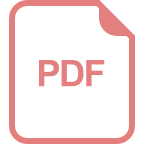
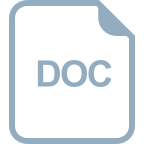
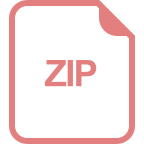
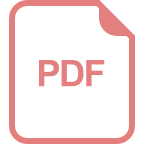
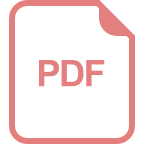
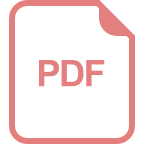
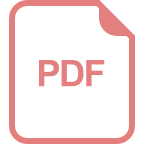
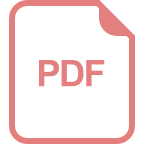
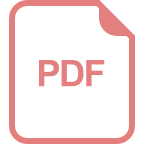
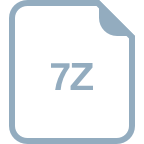
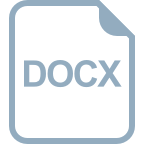
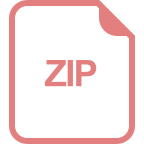
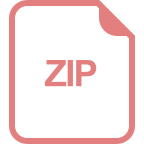