使用Java和mysql写一个空调收费系统
时间: 2024-02-22 07:54:57 浏览: 173
好的,我可以为您提供一些思路和代码示例。
首先,您需要创建一个名为"air_conditioning"的数据库,其中包含两个表:一个是"users"表,用于存储用户信息,另一个是"charges"表,用于记录用户的空调使用情况和收费信息。
以下是创建这两个表的SQL语句:
```
CREATE TABLE users (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
balance DECIMAL(10,2) NOT NULL,
PRIMARY KEY (id)
);
CREATE TABLE charges (
id INT NOT NULL AUTO_INCREMENT,
user_id INT NOT NULL,
start_time DATETIME NOT NULL,
end_time DATETIME NOT NULL,
cost DECIMAL(10,2) NOT NULL,
PRIMARY KEY (id),
FOREIGN KEY (user_id) REFERENCES users(id)
);
```
接下来,您需要编写Java代码来连接数据库并实现收费系统的功能。以下是一个简单的示例:
```java
import java.sql.*;
public class AirConditioningSystem {
private static final String DB_URL = "jdbc:mysql://localhost/air_conditioning";
private static final String DB_USER = "root";
private static final String DB_PASSWORD = "password";
public static void main(String[] args) {
try {
Connection conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
System.out.println("Connected to database");
// 用户充值
recharge(conn, 1, 100.00);
// 用户使用空调
useAirConditioning(conn, 1);
// 查询用户余额和使用记录
queryUser(conn, 1);
conn.close();
System.out.println("Disconnected from database");
} catch (SQLException e) {
e.printStackTrace();
}
}
// 用户充值
public static void recharge(Connection conn, int userId, double amount) throws SQLException {
String sql = "UPDATE users SET balance = balance + ? WHERE id = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setDouble(1, amount);
stmt.setInt(2, userId);
stmt.executeUpdate();
System.out.println("User " + userId + " has recharged " + amount + " yuan");
}
// 用户使用空调
public static void useAirConditioning(Connection conn, int userId) throws SQLException {
// 检查用户余额
String sql1 = "SELECT balance FROM users WHERE id = ?";
PreparedStatement stmt1 = conn.prepareStatement(sql1);
stmt1.setInt(1, userId);
ResultSet rs1 = stmt1.executeQuery();
if (!rs1.next()) {
System.out.println("User " + userId + " not found");
return;
}
double balance = rs1.getDouble("balance");
if (balance < 1.00) {
System.out.println("User " + userId + " has insufficient balance");
return;
}
// 记录空调使用情况和费用
String sql2 = "INSERT INTO charges (user_id, start_time, end_time, cost) VALUES (?, NOW(), NOW() + INTERVAL 1 HOUR, 1.00)";
PreparedStatement stmt2 = conn.prepareStatement(sql2);
stmt2.setInt(1, userId);
stmt2.executeUpdate();
// 扣除费用
String sql3 = "UPDATE users SET balance = balance - 1.00 WHERE id = ?";
PreparedStatement stmt3 = conn.prepareStatement(sql3);
stmt3.setInt(1, userId);
stmt3.executeUpdate();
System.out.println("User " + userId + " has used the air conditioning");
}
// 查询用户余额和使用记录
public static void queryUser(Connection conn, int userId) throws SQLException {
String sql1 = "SELECT name, balance FROM users WHERE id = ?";
PreparedStatement stmt1 = conn.prepareStatement(sql1);
stmt1.setInt(1, userId);
ResultSet rs1 = stmt1.executeQuery();
if (!rs1.next()) {
System.out.println("User " + userId + " not found");
return;
}
String name = rs1.getString("name");
double balance = rs1.getDouble("balance");
System.out.println("User " + userId + " (" + name + ") has a balance of " + balance + " yuan");
String sql2 = "SELECT start_time, end_time, cost FROM charges WHERE user_id = ?";
PreparedStatement stmt2 = conn.prepareStatement(sql2);
stmt2.setInt(1, userId);
ResultSet rs2 = stmt2.executeQuery();
while (rs2.next()) {
Timestamp startTime = rs2.getTimestamp("start_time");
Timestamp endTime = rs2.getTimestamp("end_time");
double cost = rs2.getDouble("cost");
System.out.println(" Usage: " + startTime + " - " + endTime + ", cost " + cost + " yuan");
}
}
}
```
上述代码演示了用户充值、使用空调和查询余额和使用记录的功能。您可以根据需要进行修改和扩展。
希望这可以帮助您开始编写空调收费系统。
阅读全文
相关推荐
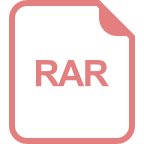
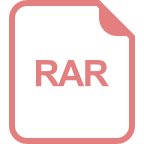
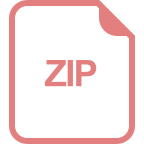
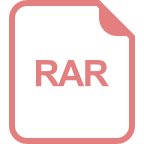
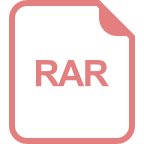
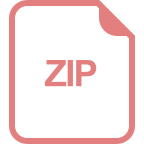
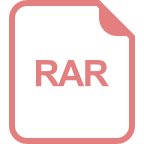
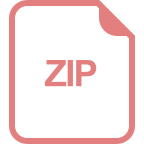
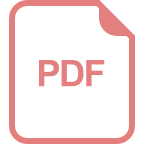
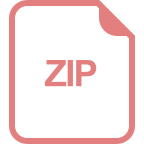
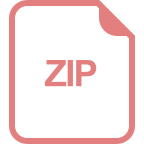
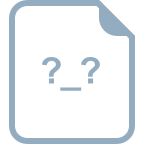
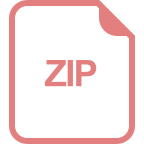
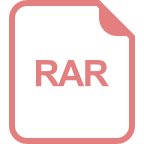
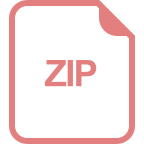
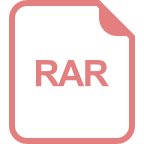