创建一个学生类(含姓名,性别,年龄等信息),从键盘上输入学生信息,把学生对象保存在指定文本文件中的指定位置。从文本文件中读取特定学生信息。实现学生信息的增加、删除、修改、插入等操作。
时间: 2024-02-06 09:10:23 浏览: 53
以下是一个Python实现的学生类代码:
```python
class Student:
def __init__(self, name, gender, age):
self.name = name
self.gender = gender
self.age = age
def __str__(self):
return f"Name: {self.name}, Gender: {self.gender}, Age: {self.age}"
```
接下来是实现增删改查的代码:
```python
import os
# 文件路径,可根据需要修改
FILE_PATH = "students.txt"
def save_student(student, file_path, position):
with open(file_path, "r+") as f:
# 定位到指定位置
f.seek(position)
# 写入学生信息
f.write(str(student))
# 移动文件指针到行末
f.seek(0, os.SEEK_END)
# 记录当前位置,以备下次写入
position = f.tell()
return position
def read_student(name, file_path):
with open(file_path, "r") as f:
for line in f:
# 按行读取文件,查找特定学生信息
if name in line:
# 如果找到,返回学生对象
return eval(line)
# 如果未找到,返回None
return None
def add_student(student, file_path):
with open(file_path, "a") as f:
# 追加学生信息
f.write(str(student) + "\n")
def remove_student(name, file_path):
with open(file_path, "r") as f:
lines = f.readlines()
with open(file_path, "w") as f:
for line in lines:
# 按行读取文件,删除特定学生信息
if name not in line:
# 如果不是目标学生,写入文件
f.write(line)
def update_student(student, file_path):
with open(file_path, "r") as f:
lines = f.readlines()
with open(file_path, "w") as f:
for line in lines:
# 按行读取文件,更新特定学生信息
if student.name in line:
# 如果是目标学生,写入更新后的信息
f.write(str(student) + "\n")
else:
# 如果不是目标学生,写入原来的信息
f.write(line)
def insert_student(student, file_path, position):
with open(file_path, "r+") as f:
# 定位到指定位置
f.seek(position)
# 读取后面的内容
rest = f.read()
# 回到插入点
f.seek(position)
# 写入学生信息
f.write(str(student) + "\n")
# 写入后面的内容
f.write(rest)
# 记录当前位置,以备下次写入
position = f.tell()
return position
```
最后是一个简单的使用样例:
```python
if __name__ == "__main__":
# 从键盘输入学生信息
name = input("Name: ")
gender = input("Gender: ")
age = input("Age: ")
student = Student(name, gender, age)
# 保存学生信息到文件
file_path = FILE_PATH
position = save_student(student, file_path, 0)
# 读取特定学生信息
name = input("Name to search: ")
student = read_student(name, file_path)
if student is not None:
print(student)
else:
print("Student not found.")
# 增加学生信息
name = input("Name: ")
gender = input("Gender: ")
age = input("Age: ")
student = Student(name, gender, age)
add_student(student, file_path)
# 删除学生信息
name = input("Name to remove: ")
remove_student(name, file_path)
# 更新学生信息
name = input("Name to update: ")
gender = input("Gender: ")
age = input("Age: ")
student = Student(name, gender, age)
update_student(student, file_path)
# 插入学生信息
name = input("Name: ")
gender = input("Gender: ")
age = input("Age: ")
student = Student(name, gender, age)
position = insert_student(student, file_path, position)
```
注意,这里的保存方式是将学生对象直接转换为字符串,并写入文件。如果需要更复杂的保存方式,可以考虑使用JSON、XML等格式。另外,这里的读写文件方式使用了Python内置的open函数,也可以考虑使用第三方库如pandas来处理文件。
阅读全文
相关推荐
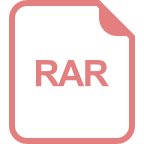
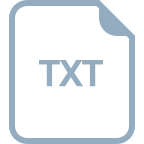
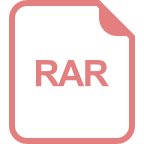



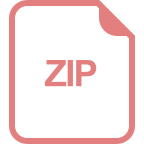
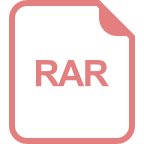
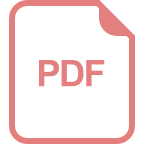
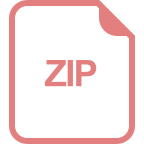
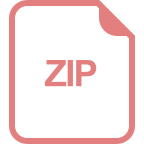