如何使用eclipse实现学生选课系统,具体代码加注释
时间: 2024-03-17 17:42:04 浏览: 55
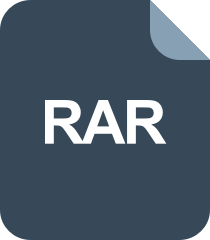
学生选课系统源代码asp.net + c# + access

实现学生选课系统需要进行数据库设计、Web框架选择、前端页面设计、后端Java代码编写等多个步骤,这里提供一个简单的实现思路和代码示例,供参考。
1. 数据库设计
首先需要设计数据库表结构,包括学生信息、课程信息、教师信息、选课信息等。可以使用MySQL或其他关系型数据库。
2. Web框架选择
这里以SpringMVC为例,使用Maven进行依赖管理。
3. 前端页面设计
使用HTML、CSS和JavaScript进行前端页面设计,可以使用Bootstrap等前端框架加快开发进度。
4. 后端Java代码编写
下面是简单的Java代码示例,实现学生选课系统的基本功能。
首先是学生信息的JavaBean类:
```java
public class Student {
private int id;
private String name;
private String password;
// 其他属性和方法省略
}
```
然后是课程信息的JavaBean类:
```java
public class Course {
private int id;
private String name;
private int teacherId;
private int capacity;
// 其他属性和方法省略
}
```
再是教师信息的JavaBean类:
```java
public class Teacher {
private int id;
private String name;
private String password;
// 其他属性和方法省略
}
```
最后是选课信息的JavaBean类:
```java
public class CourseSelection {
private int id;
private int studentId;
private int courseId;
// 其他属性和方法省略
}
```
接下来是控制器类,实现学生登录、选课、退课等功能:
```java
@Controller
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@Autowired
private CourseService courseService;
@Autowired
private CourseSelectionService courseSelectionService;
@RequestMapping("/login")
public String login(@RequestParam("name") String name, @RequestParam("password") String password, HttpSession session) {
Student student = studentService.getStudentByName(name);
if (student != null && student.getPassword().equals(password)) {
session.setAttribute("student", student);
return "redirect:/course/list";
} else {
return "login";
}
}
@RequestMapping("/logout")
public String logout(HttpSession session) {
session.removeAttribute("student");
return "redirect:/";
}
@RequestMapping("/choose")
public String choose(@RequestParam("courseId") int courseId, HttpSession session) {
Student student = (Student) session.getAttribute("student");
if (student == null) {
return "redirect:/";
}
Course course = courseService.getCourseById(courseId);
if (course == null) {
return "redirect:/course/list";
}
if (course.getCapacity() <= 0) {
return "redirect:/course/list";
}
CourseSelection courseSelection = new CourseSelection();
courseSelection.setStudentId(student.getId());
courseSelection.setCourseId(courseId);
courseSelectionService.addCourseSelection(courseSelection);
course.setCapacity(course.getCapacity() - 1);
courseService.updateCourse(course);
return "redirect:/course/list";
}
@RequestMapping("/drop")
public String drop(@RequestParam("courseId") int courseId, HttpSession session) {
Student student = (Student) session.getAttribute("student");
if (student == null) {
return "redirect:/";
}
Course course = courseService.getCourseById(courseId);
if (course == null) {
return "redirect:/course/list";
}
CourseSelection courseSelection = courseSelectionService.getCourseSelectionByStudentAndCourse(student.getId(), courseId);
if (courseSelection == null) {
return "redirect:/course/list";
}
courseSelectionService.deleteCourseSelection(courseSelection.getId());
course.setCapacity(course.getCapacity() + 1);
courseService.updateCourse(course);
return "redirect:/course/list";
}
}
```
这里使用了SpringMVC的注解,例如@Controller、@RequestMapping等。控制器类中包含了学生登录、选课、退课等功能的实现,具体实现过程中需要依赖Service层和DAO层进行数据库操作。
下面是Service层和DAO层的代码示例,以学生信息为例:
Service层:
```java
@Service
public class StudentService {
@Autowired
private StudentDao studentDao;
public Student getStudentByName(String name) {
return studentDao.getStudentByName(name);
}
}
```
DAO层:
```java
@Repository
public interface StudentDao {
Student getStudentByName(String name);
}
```
这里使用了Spring的注解,例如@Service、@Repository等,依赖MyBatis进行数据库操作。
以上是简单的Java代码示例,实现了学生选课系统的基本功能,具体实现过程中还需要根据具体需求进行调整和优化。
阅读全文
相关推荐
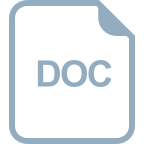
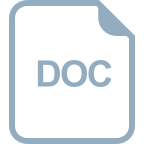
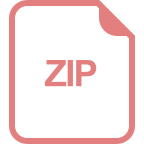
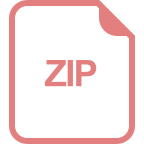
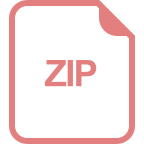
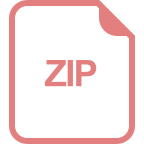
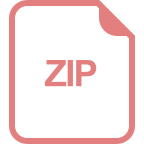
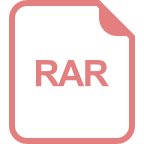
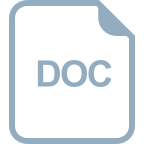
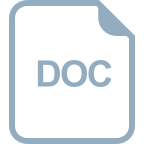
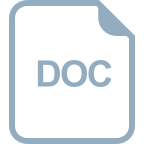
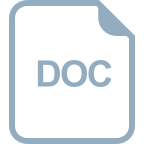
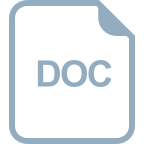
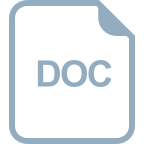
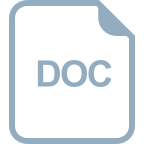
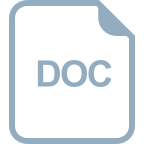
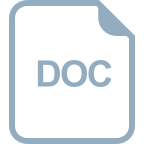