SpringBoot加入Guava Cache实现本地缓存代码实例
时间: 2023-12-15 20:24:21 浏览: 39
以下是一个简单的Spring Boot应用程序,使用Guava Cache实现本地缓存:
首先,需要在pom.xml文件中添加依赖:
```
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>28.0-jre</version>
</dependency>
```
然后,创建一个CacheManager bean来管理缓存:
```
@Configuration
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new GuavaCacheManager("myCache");
}
}
```
在上面的代码中,我们使用GuavaCacheManager创建一个名为“myCache”的缓存管理器。
接下来,创建一个Service bean来使用缓存:
```
@Service
public class MyService {
@Autowired
private CacheManager cacheManager;
public String getData(String key) {
Cache cache = cacheManager.getCache("myCache");
Cache.ValueWrapper valueWrapper = cache.get(key);
if (valueWrapper != null) {
return (String) valueWrapper.get();
} else {
String data = getDataFromDatabase(key);
cache.put(key, data);
return data;
}
}
private String getDataFromDatabase(String key) {
// 从数据库获取数据
return "data for " + key;
}
}
```
在上面的代码中,我们注入了CacheManager bean,并使用它来获取名为“myCache”的缓存。如果缓存中不存在所需的数据,我们从数据库中获取数据并将其放入缓存中。
现在,我们可以测试该应用程序是否正常工作:
```
@RestController
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/data")
public String getData(@RequestParam String key) {
return myService.getData(key);
}
}
```
在上面的代码中,我们注入了MyService bean,并在HTTP GET请求中使用它来获取数据。现在,我们可以使用curl或浏览器访问http://localhost:8080/data?key=test,应该会返回“data for test”。如果我们再次访问http://localhost:8080/data?key=test,应该会返回缓存中的数据,而不是从数据库中获取数据。
这就是使用Guava Cache在Spring Boot应用程序中实现本地缓存的简单示例。
相关推荐
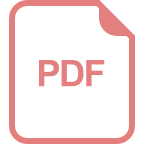
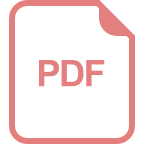
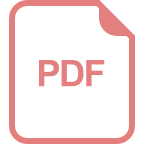
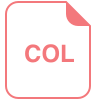











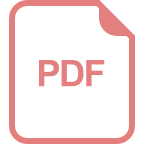
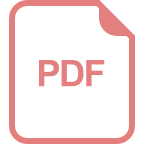
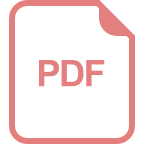