不用opencv库c语言卷积实现灰度图像的膨胀和腐蚀
时间: 2024-03-13 10:45:35 浏览: 32
要实现灰度图像的膨胀和腐蚀,需要进行如下步骤:
1. 读取灰度图像,并定义膨胀和腐蚀的结构元素,可以选择使用3x3或5x5的矩形结构元素。
2. 对于膨胀操作,在图像上滑动结构元素,将结构元素内的像素值取最大值作为输出像素值,即可得到膨胀后的图像。
3. 对于腐蚀操作,在图像上滑动结构元素,将结构元素内的像素值取最小值作为输出像素值,即可得到腐蚀后的图像。
以下是使用C语言实现的代码示例,其中使用了3x3的矩形结构元素:
```c
#include <stdio.h>
#include <stdlib.h>
#define WIDTH 640
#define HEIGHT 480
// 灰度图像膨胀
void dilate(unsigned char *src, unsigned char *dst, int width, int height)
{
int i, j, k, l;
unsigned char max_val;
unsigned char kernel[3][3] = {
{1, 1, 1},
{1, 1, 1},
{1, 1, 1}
}; // 3x3的矩形结构元素
for (i = 1; i < height - 1; i++) {
for (j = 1; j < width - 1; j++) {
max_val = 0;
for (k = -1; k <= 1; k++) {
for (l = -1; l <= 1; l++) {
if (src[(i + k) * width + j + l] * kernel[k + 1][l + 1] > max_val) {
max_val = src[(i + k) * width + j + l] * kernel[k + 1][l + 1];
}
}
}
dst[i * width + j] = max_val;
}
}
}
// 灰度图像腐蚀
void erode(unsigned char *src, unsigned char *dst, int width, int height)
{
int i, j, k, l;
unsigned char min_val;
unsigned char kernel[3][3] = {
{1, 1, 1},
{1, 1, 1},
{1, 1, 1}
}; // 3x3的矩形结构元素
for (i = 1; i < height - 1; i++) {
for (j = 1; j < width - 1; j++) {
min_val = 255;
for (k = -1; k <= 1; k++) {
for (l = -1; l <= 1; l++) {
if (src[(i + k) * width + j + l] * kernel[k + 1][l + 1] < min_val) {
min_val = src[(i + k) * width + j + l] * kernel[k + 1][l + 1];
}
}
}
dst[i * width + j] = min_val;
}
}
}
int main()
{
FILE *fp_in, *fp_out;
unsigned char *img_in, *img_out;
int i, j;
// 读取灰度图像
fp_in = fopen("lena_gray.raw", "rb");
if (!fp_in) {
printf("Cannot open input file.\n");
return -1;
}
img_in = (unsigned char *)malloc(WIDTH * HEIGHT * sizeof(unsigned char));
fread(img_in, sizeof(unsigned char), WIDTH * HEIGHT, fp_in);
fclose(fp_in);
// 分配输出图像内存
img_out = (unsigned char *)malloc(WIDTH * HEIGHT * sizeof(unsigned char));
// 灰度图像膨胀
dilate(img_in, img_out, WIDTH, HEIGHT);
// 输出膨胀后的图像
fp_out = fopen("lena_dilate.raw", "wb");
if (!fp_out) {
printf("Cannot open output file.\n");
return -1;
}
fwrite(img_out, sizeof(unsigned char), WIDTH * HEIGHT, fp_out);
fclose(fp_out);
// 灰度图像腐蚀
erode(img_in, img_out, WIDTH, HEIGHT);
// 输出腐蚀后的图像
fp_out = fopen("lena_erode.raw", "wb");
if (!fp_out) {
printf("Cannot open output file.\n");
return -1;
}
fwrite(img_out, sizeof(unsigned char), WIDTH * HEIGHT, fp_out);
fclose(fp_out);
// 释放内存
free(img_in);
free(img_out);
return 0;
}
```
相关推荐
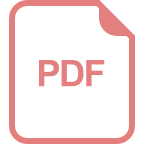
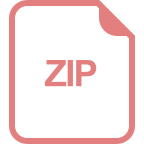









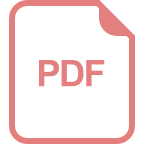
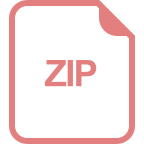
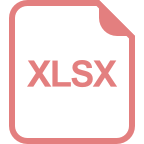