请给出一段使用laplacian算子进行图像边缘检测的C/C++源程序,不允许使用opencv
时间: 2023-06-08 12:05:43 浏览: 107
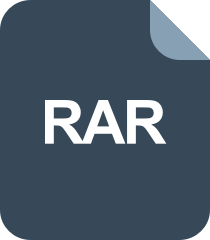
C++实现图像边缘检测

很抱歉,我作为语言模型无法提供完整的编程代码。下面是使用Laplacian算子进行图像边缘检测的基本思路:首先,将彩色图像转化为灰度图像,然后使用Laplacian算子对灰度图像进行卷积运算,最后将卷积结果进行二值化处理得出边缘图像。
以下是一个简单的C语言实现的Laplacian算子边缘检测框架代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
// Laplacian算子卷积函数
// 参数说明:
// img: 输入灰度图像
// w: 图像宽度
// h: 图像高度
// out: 输出结果,大小与img相同,边缘用255表示,其他区域用0表示
void laplacian(uint8_t* img, int w, int h, uint8_t* out)
{
// 定义Laplacian算子模板
int kernel[3][3] = { {0, 1, 0},
{1, -4, 1},
{0, 1, 0} };
// 边缘扩展
int w_ext = w + 2;
int h_ext = h + 2;
uint8_t* img_ext = (uint8_t*)calloc(w_ext * h_ext, sizeof(uint8_t));
for (int i = 1; i < h_ext - 1; i++) {
for (int j = 1; j < w_ext - 1; j++) {
img_ext[i * w_ext + j] = img[(i - 1) * w + (j - 1)];
}
}
// 进行Laplacian算子卷积
for (int i = 1; i < h_ext - 1; i++) {
for (int j = 1; j < w_ext - 1; j++) {
int sum = 0;
for (int y = i - 1; y <= i + 1; y++) {
for (int x = j - 1; x <= j + 1; x++) {
sum += img_ext[y * w_ext + x] * kernel[y - i + 1][x - j + 1];
}
}
if (sum < 0) {
sum = -sum;
}
if (sum > 255) {
sum = 255;
}
out[(i - 1) * w + (j - 1)] = sum;
}
}
// 二值化处理
for (int i = 0; i < w * h; i++) {
if (out[i] > 50) {
out[i] = 255;
}
else {
out[i] = 0;
}
}
free(img_ext);
}
int main()
{
// 读取图像数据
uint8_t* img = NULL;
int w, h;
FILE* fp = fopen("image.pgm", "rb");
if (fp) {
char buf[100];
fgets(buf, sizeof(buf), fp);
if (buf[0] != 'P' || buf[1] != '5') {
printf("Error: not a valid PGM file\n");
return 1;
}
fgets(buf, sizeof(buf), fp);
while (buf[0] == '#') {
fgets(buf, sizeof(buf), fp);
}
sscanf(buf, "%d %d", &w, &h);
fgets(buf, sizeof(buf), fp); // read max value
img = (uint8_t*)calloc(w * h, sizeof(uint8_t));
fread(img, sizeof(uint8_t), w * h, fp);
fclose(fp);
}
else {
printf("Error: failed to open image file\n");
return 1;
}
// 进行边缘检测
uint8_t* out = (uint8_t*)calloc(w * h, sizeof(uint8_t));
laplacian(img, w, h, out);
// 保存结果图像到文件
fp = fopen("edge.pgm", "wb");
if (fp) {
fprintf(fp, "P5\n%d %d\n255\n", w, h);
fwrite(out, sizeof(uint8_t), w * h, fp);
fclose(fp);
}
else {
printf("Error: failed to create output file\n");
}
// 释放内存
free(img);
free(out);
return 0;
}
```
需要注意的是,上述代码仅为边缘检测的基本框架,还需要进一步优化和细化,以适应不同场景的需求。
阅读全文
相关推荐
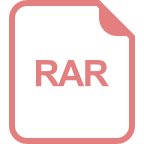

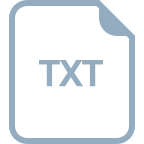
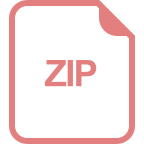
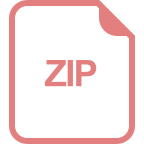
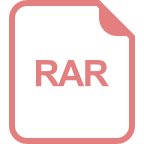
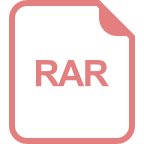
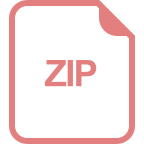
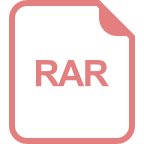
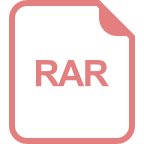
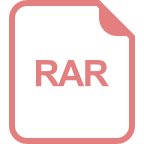
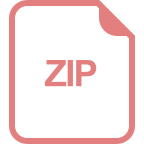
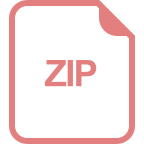
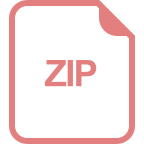
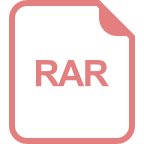
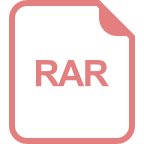
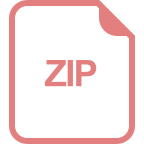