C语言返回值为结构体的函数
时间: 2025-01-04 19:32:42 浏览: 17
### C语言中定义和使用返回结构体的函数
在C语言中,可以通过函数来返回整个结构体对象。这使得程序能够更加灵活地处理复杂的数据类型。
#### 函数返回结构体的方式
当希望从函数获取一个完整的结构体实例时,可以直接让该函数返回这个类型的值。下面是一个具体的例子:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体并创建别名
typedef struct {
char name[20];
int age;
float score;
} Student;
// 声明一个返回Student类型数据的函数
Student create_student(const char *name, int age, float score);
int main() {
// 调用create_student函数获得新创建的学生记录
Student stu = create_student("Alice", 18, 95.5);
printf("Name: %s\nAge: %d\nScore: %.2f\n", stu.name, stu.age, stu.score);
}
// 实现create_student函数
Student create_student(const char *name, int age, float score) {
Student temp_stu;
strcpy(temp_stu.name, name); // 使用strcpy复制字符串到数组
temp_stu.age = age; // 设置年龄属性
temp_stu.score = score; // 设定成绩属性
return temp_stu; // 返回构建好的结构体实例
}
```
上述代码展示了如何定义一个名为`create_student` 的函数,它接收三个参数用于初始化一个新的 `Student` 结构体,并将其作为结果返回给调用者[^1]。
需要注意的是,在某些情况下,如果结构体内存占用较大,则可能更倾向于通过指针传递结构体以提高性能[^3]。然而对于小型结构体来说,直接按值返回通常是安全且高效的方案。
阅读全文
相关推荐









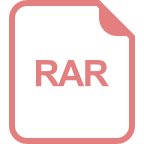








