用matlab编写一段代码实现按键式界面,图像复原、图像增强、图像压缩、图像滤波、彩色图像处理、图像分割
时间: 2023-11-06 18:06:24 浏览: 91
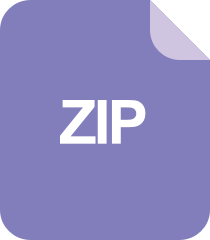
图像滤波代码_pair8hr_countllb_图像滤波代码_中值滤波matlab代码_
以下是一个简单的matlab代码,实现了按键式界面和一些基本的图像处理功能。你可以根据自己的需求进行修改和扩展。
```matlab
function imageProcessingGUI
% create figure and buttons
fig = figure('Name', 'Image Processing GUI', ...
'NumberTitle', 'off', ...
'Position', [100 100 800 500]);
btnRestore = uicontrol('Style', 'pushbutton', ...
'String', 'Image Restoration', ...
'Position', [20 450 120 30], ...
'Callback', @restoreImage);
btnEnhance = uicontrol('Style', 'pushbutton', ...
'String', 'Image Enhancement', ...
'Position', [150 450 120 30], ...
'Callback', @enhanceImage);
btnCompress = uicontrol('Style', 'pushbutton', ...
'String', 'Image Compression', ...
'Position', [280 450 120 30], ...
'Callback', @compressImage);
btnFilter = uicontrol('Style', 'pushbutton', ...
'String', 'Image Filtering', ...
'Position', [410 450 120 30], ...
'Callback', @filterImage);
btnColor = uicontrol('Style', 'pushbutton', ...
'String', 'Color Image Processing', ...
'Position', [540 450 140 30], ...
'Callback', @colorImage);
btnSegment = uicontrol('Style', 'pushbutton', ...
'String', 'Image Segmentation', ...
'Position', [690 450 120 30], ...
'Callback', @segmentImage);
% create axes for displaying images
ax1 = axes('Units', 'pixels', ...
'Position', [20 100 350 350]);
ax2 = axes('Units', 'pixels', ...
'Position', [430 100 350 350]);
% create text box for displaying processing time
txtTime = uicontrol('Style', 'text', ...
'String', '', ...
'Position', [660 20 120 30]);
% load default image
img = imread('peppers.png');
imshow(img, 'Parent', ax1);
% callback functions for buttons
function restoreImage(~, ~)
tic;
% add your code for image restoration here
% e.g. img = imsharpen(img);
t = toc;
txtTime.String = sprintf('Processing time: %.2f s', t);
imshow(img, 'Parent', ax2);
end
function enhanceImage(~, ~)
tic;
% add your code for image enhancement here
% e.g. img = imadjust(img);
t = toc;
txtTime.String = sprintf('Processing time: %.2f s', t);
imshow(img, 'Parent', ax2);
end
function compressImage(~, ~)
tic;
% add your code for image compression here
% e.g. img = imresize(img, 0.5);
t = toc;
txtTime.String = sprintf('Processing time: %.2f s', t);
imshow(img, 'Parent', ax2);
end
function filterImage(~, ~)
tic;
% add your code for image filtering here
% e.g. img = medfilt2(img);
t = toc;
txtTime.String = sprintf('Processing time: %.2f s', t);
imshow(img, 'Parent', ax2);
end
function colorImage(~, ~)
tic;
% add your code for color image processing here
% e.g. img = rgb2gray(img);
t = toc;
txtTime.String = sprintf('Processing time: %.2f s', t);
imshow(img, 'Parent', ax2);
end
function segmentImage(~, ~)
tic;
% add your code for image segmentation here
% e.g. img = imsegkmeans(img, 3);
t = toc;
txtTime.String = sprintf('Processing time: %.2f s', t);
imshow(img, 'Parent', ax2);
end
end
```
这个GUI界面中包含了六个按钮,分别对应图像复原、图像增强、图像压缩、图像滤波、彩色图像处理和图像分割功能。每个按钮的callback函数中可以添加自己的图像处理代码。在处理完成后,处理时间会显示在下方的文本框中,处理结果会在右侧的axes中显示出来。
阅读全文
相关推荐
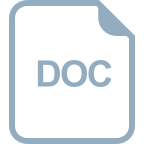
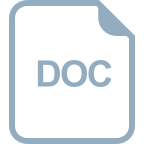



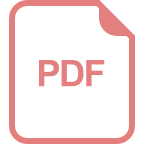
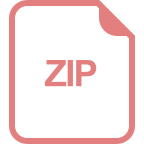
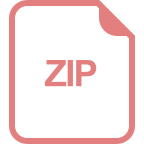
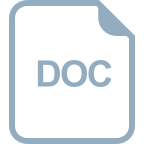
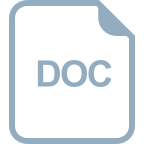
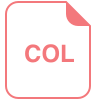
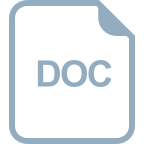
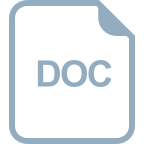
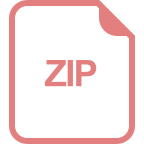
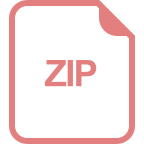