C++编程实现单目标优化问题
时间: 2023-06-25 16:05:28 浏览: 119
单目标优化问题是指在给定的约束条件下,寻找一个最优的目标函数值。C++可以通过以下步骤实现单目标优化问题:
1. 定义目标函数:首先需要定义一个目标函数,其输入参数为优化变量,输出为目标函数值。
2. 设置约束条件:根据实际问题需要,设置一定的约束条件,如变量范围、等式约束、不等式约束等。
3. 选择优化算法:根据实际问题需要,选择合适的优化算法,如梯度下降法、遗传算法、粒子群算法等。
4. 实现优化算法:根据选择的优化算法,实现相应的代码。
5. 调用优化函数:将目标函数和约束条件传入优化函数中,调用优化函数进行求解。
下面是一个简单的例子,使用遗传算法求解单目标优化问题:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
#include <algorithm>
#include <random>
using namespace std;
// 目标函数
double objective(vector<double>& x)
{
return pow(x[0], 2) + pow(x[1], 2) - 0.3 * cos(3 * M_PI * x[0]) - 0.4 * cos(4 * M_PI * x[1]) + 0.7;
}
// 约束条件
bool constraint(vector<double>& x)
{
if (x[0] < -1 || x[0] > 1 || x[1] < -1 || x[1] > 1)
return false;
return true;
}
// 交叉操作
void crossover(vector<double>& parent1, vector<double>& parent2, vector<double>& child)
{
int size = parent1.size();
for (int i = 0; i < size; ++i) {
if (rand() % 2 == 0)
child[i] = parent1[i];
else
child[i] = parent2[i];
}
}
// 变异操作
void mutate(vector<double>& x)
{
int size = x.size();
int index = rand() % size;
double delta = ((double)rand() / RAND_MAX - 0.5) * 0.1;
x[index] += delta;
}
// 遗传算法优化函数
vector<double> ga_optimization(int pop_size, int max_iter, double mutation_rate)
{
int dim = 2; // 优化变量维度
vector<vector<double>> population(pop_size, vector<double>(dim)); // 种群
vector<double> fitness(pop_size); // 适应度
vector<double> best(dim); // 最佳个体
double best_fitness = numeric_limits<double>::max(); // 最佳适应度
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<double> dis(-1, 1);
// 初始化种群
for (int i = 0; i < pop_size; ++i) {
for (int j = 0; j < dim; ++j) {
population[i][j] = dis(gen);
}
if (!constraint(population[i]))
fitness[i] = numeric_limits<double>::max();
else
fitness[i] = objective(population[i]);
if (fitness[i] < best_fitness) {
best_fitness = fitness[i];
best = population[i];
}
}
// 迭代优化
for (int iter = 0; iter < max_iter; ++iter) {
// 选择操作
vector<vector<double>> parents(2, vector<double>(dim));
for (int i = 0; i < 2; ++i) {
uniform_int_distribution<int> dis(0, pop_size - 1);
int index1 = dis(gen);
int index2 = dis(gen);
if (fitness[index1] < fitness[index2])
parents[i] = population[index1];
else
parents[i] = population[index2];
}
// 交叉操作
vector<double> child(dim);
crossover(parents[0], parents[1], child);
// 变异操作
uniform_real_distribution<double> dis(0, 1);
if (dis(gen) < mutation_rate)
mutate(child);
// 更新种群
if (!constraint(child))
fitness[pop_size - 1] = numeric_limits<double>::max();
else
fitness[pop_size - 1] = objective(child);
population[pop_size - 1] = child;
for (int i = 0; i < pop_size - 1; ++i) {
if (fitness[i] < fitness[i + 1]) {
swap(fitness[i], fitness[i + 1]);
swap(population[i], population[i + 1]);
}
}
// 更新最优个体
if (fitness[0] < best_fitness) {
best_fitness = fitness[0];
best = population[0];
}
}
return best;
}
int main()
{
int pop_size = 100; // 种群大小
int max_iter = 100; // 最大迭代次数
double mutation_rate = 0.1; // 变异率
vector<double> result = ga_optimization(pop_size, max_iter, mutation_rate);
cout << "Optimal solution: x1 = " << result[0] << ", x2 = " << result[1] << endl;
cout << "Objective function value: " << objective(result) << endl;
return 0;
}
```
在上述代码中,我们定义了一个目标函数和一个约束条件,然后使用遗传算法进行优化。具体来说,首先初始化一个种群,然后进行一定次数的迭代,每次迭代进行选择、交叉和变异操作,更新种群,最后得到最优解。在遗传算法中,我们使用了轮盘赌选择算法、单点交叉和随机变异。
阅读全文
相关推荐
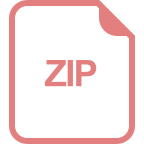
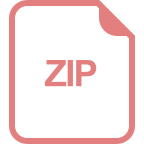
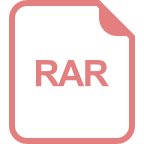
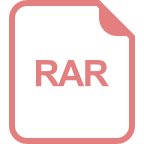
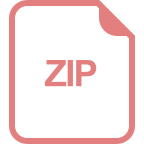
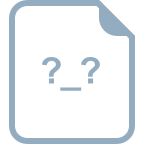
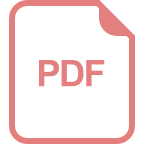
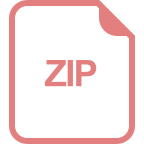
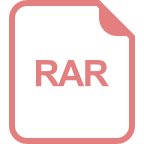
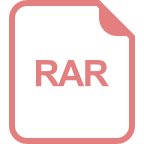
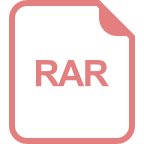
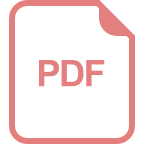
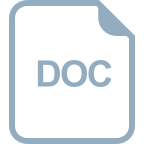
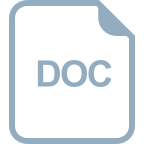