修改DCGAN代码为输入图像和输出图像的尺寸为256*256
时间: 2024-05-14 11:14:54 浏览: 21
要修改 DCGAN 代码使得输入图像和输出图像的尺寸为 256x256,需要在生成器和判别器中进行以下修改:
1. 生成器中,将输入的随机噪声向量的长度从 100 扩展到 4096,以适应更大的图像尺寸。同时,在卷积层和反卷积层中,将卷积核和反卷积核的大小从 4 改为 5,并且将步长从 2 改为 1。
```
class Generator(nn.Module):
def __init__(self, nz=4096, ngf=64, nc=3):
super(Generator, self).__init__()
self.nz = nz
self.ngf = ngf
self.nc = nc
self.fc = nn.Linear(nz, 4 * 4 * ngf * 16)
self.bn1 = nn.BatchNorm2d(ngf * 16)
self.relu = nn.ReLU(True)
self.conv1 = nn.ConvTranspose2d(ngf * 16, ngf * 8, 5, 1, 0, bias=False)
self.bn2 = nn.BatchNorm2d(ngf * 8)
self.conv2 = nn.ConvTranspose2d(ngf * 8, ngf * 4, 5, 1, 0, bias=False)
self.bn3 = nn.BatchNorm2d(ngf * 4)
self.conv3 = nn.ConvTranspose2d(ngf * 4, ngf * 2, 5, 1, 0, bias=False)
self.bn4 = nn.BatchNorm2d(ngf * 2)
self.conv4 = nn.ConvTranspose2d(ngf * 2, nc, 5, 1, 0, bias=False)
self.tanh = nn.Tanh()
def forward(self, input):
x = self.fc(input)
x = x.view(-1, self.ngf * 16, 4, 4)
x = self.bn1(x)
x = self.relu(x)
x = self.conv1(x)
x = self.bn2(x)
x = self.relu(x)
x = self.conv2(x)
x = self.bn3(x)
x = self.relu(x)
x = self.conv3(x)
x = self.bn4(x)
x = self.relu(x)
x = self.conv4(x)
output = self.tanh(x)
return output
```
2. 判别器中,将输入图像和输出图像的大小从 64 改为 256,并且在卷积层和全连接层中,将卷积核和全连接层的大小从 4 改为 5。
```
class Discriminator(nn.Module):
def __init__(self, ndf=64, nc=3):
super(Discriminator, self).__init__()
self.ndf = ndf
self.nc = nc
self.conv1 = nn.Conv2d(nc, ndf, 5, 1, 0, bias=False)
self.relu = nn.LeakyReLU(0.2, inplace=True)
self.conv2 = nn.Conv2d(ndf, ndf * 2, 5, 1, 0, bias=False)
self.bn2 = nn.BatchNorm2d(ndf * 2)
self.conv3 = nn.Conv2d(ndf * 2, ndf * 4, 5, 1, 0, bias=False)
self.bn3 = nn.BatchNorm2d(ndf * 4)
self.conv4 = nn.Conv2d(ndf * 4, ndf * 8, 5, 1, 0, bias=False)
self.bn4 = nn.BatchNorm2d(ndf * 8)
self.fc = nn.Linear(ndf * 8 * 5 * 5, 1)
def forward(self, input):
x = self.conv1(input)
x = self.relu(x)
x = self.conv2(x)
x = self.bn2(x)
x = self.relu(x)
x = self.conv3(x)
x = self.bn3(x)
x = self.relu(x)
x = self.conv4(x)
x = self.bn4(x)
x = self.relu(x)
x = x.view(-1, self.ndf * 8 * 5 * 5)
output = self.fc(x)
return output.view(-1, 1).squeeze(1)
```
相关推荐
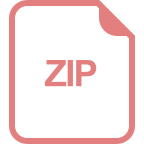














