vue axios 调用 java webservice soap 接口
时间: 2023-10-29 13:06:31 浏览: 212
可以使用 axios 发送 POST 请求来调用 Java WebService SOAP 接口。首先需要了解 SOAP 协议及其请求格式,然后可以按照以下步骤进行调用。
1. 安装 axios
可以使用 npm 安装 axios:
```
npm install axios --save
```
2. 构造 SOAP 请求
构造 SOAP 请求的格式如下:
```xml
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ser="http://service.example.com">
<soapenv:Header/>
<soapenv:Body>
<ser:methodName>
<!--Optional:-->
<arg0>?</arg0>
<!--Optional:-->
<arg1>?</arg1>
</ser:methodName>
</soapenv:Body>
</soapenv:Envelope>
```
其中,`ser:methodName` 为要调用的 Java WebService 方法名,`arg0`、`arg1` 等为方法参数,可以根据实际情况进行修改。
3. 发送 SOAP 请求
使用 axios 发送 SOAP 请求的代码如下:
```javascript
import axios from 'axios'
const url = 'http://example.com/soap/service' // WebService 地址
const action = 'http://service.example.com/methodName' // SOAPAction
const xml = `
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ser="http://service.example.com">
<soapenv:Header/>
<soapenv:Body>
<ser:methodName>
<!--Optional:-->
<arg0>?</arg0>
<!--Optional:-->
<arg1>?</arg1>
</ser:methodName>
</soapenv:Body>
</soapenv:Envelope>
` // SOAP 请求体
axios.post(url, xml, {
headers: {
'Content-Type': 'text/xml;charset=UTF-8',
'SOAPAction': action
}
}).then(response => {
console.log(response.data)
}).catch(error => {
console.log(error)
})
```
其中,`url` 为 WebService 地址,`action` 为 SOAPAction,需要根据实际情况进行修改。`xml` 为 SOAP 请求体,需要根据实际情况进行修改。在发送请求时,需要指定 `Content-Type` 为 `text/xml;charset=UTF-8`,并在请求头中加入 `SOAPAction`。
4. 解析响应
Java WebService 返回的响应也是 SOAP 格式的,需要进行解析。可以使用第三方库 `xml2js` 将响应转换为 JavaScript 对象,代码如下:
```javascript
import axios from 'axios'
import { parseString } from 'xml2js'
const url = 'http://example.com/soap/service' // WebService 地址
const action = 'http://service.example.com/methodName' // SOAPAction
const xml = `
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ser="http://service.example.com">
<soapenv:Header/>
<soapenv:Body>
<ser:methodName>
<!--Optional:-->
<arg0>?</arg0>
<!--Optional:-->
<arg1>?</arg1>
</ser:methodName>
</soapenv:Body>
</soapenv:Envelope>
` // SOAP 请求体
axios.post(url, xml, {
headers: {
'Content-Type': 'text/xml;charset=UTF-8',
'SOAPAction': action
}
}).then(response => {
parseString(response.data, (error, result) => {
if (error) {
console.log(error)
} else {
console.log(result)
}
})
}).catch(error => {
console.log(error)
})
```
在响应中,可以通过 `result.Body` 获取到 Java WebService 返回的实际内容,需要根据实际情况进行解析。
阅读全文
相关推荐


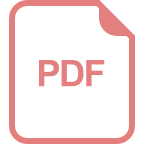
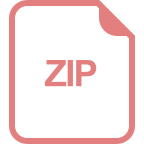
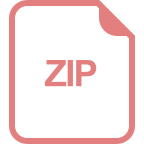
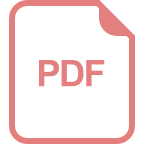
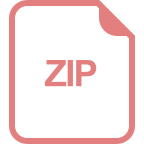
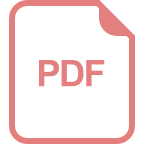
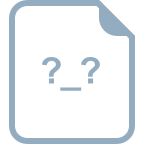
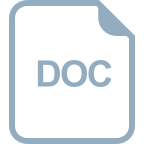
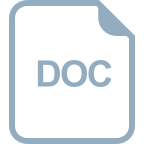
