register address reg_access field field_access reset_value bitpos_end bitpos_start function chnl0_ctrl 0x00 RW chnl_en RW 0x1 0 0 channel enable prio_level RW 0x3 2 1 priority level pkt_len RW 0x0 5 3 packet length reserved RO 0x0 31 6 reserved chnl1_ctrl 0x04 RW chnl_en RW 0x1 0 0 channel enable prio_level RW 0x3 2 1 priority level pkt_len RW 0x0 5 3 packet length reserved RO 0x0 31 6 reserved chnl2_ctrl 0x08 RW chnl_en RW 0x1 0 0 channel enable prio_level RW 0x3 2 1 priority level pkt_len RW 0x0 5 3 packet length reserved RO 0x0 31 6 reserved chnl0_stat 0x10 RO fifo_avail RO 0x20 7 0 fifo available storage reserved RO 0x0 31 8 reserved chnl1_stat 0x14 RO fifo_avail RO 0x20 7 0 fifo available storage reserved RO 0x0 31 8 reserved chnl2_stat 0x18 RO fifo_avail RO 0x20 7 0 fifo available storage reserved RO 0x0 31 8 reserved
时间: 2024-02-07 10:03:12 浏览: 32
根据提供的信息,我们可以将Excel表格中的信息转化为如下的UVM RAL模板:
```systemverilog
// 寄存器地址定义
localparam int REG_ADDR_CHNL0_CTRL = 32'h00000000;
localparam int REG_ADDR_CHNL1_CTRL = 32'h00000004;
localparam int REG_ADDR_CHNL2_CTRL = 32'h00000008;
localparam int REG_ADDR_CHNL0_STAT = 32'h00000010;
localparam int REG_ADDR_CHNL1_STAT = 32'h00000014;
localparam int REG_ADDR_CHNL2_STAT = 32'h00000018;
// 寄存器访问权限定义
typedef enum bit [1:0] {
REG_ACCESS_RW = 2'b00,
REG_ACCESS_RO = 2'b01,
REG_ACCESS_WO = 2'b10,
REG_ACCESS_RSVD = 2'b11
} reg_access_e;
// 字段访问权限定义
typedef enum bit [1:0] {
FIELD_ACCESS_RW = 2'b00,
FIELD_ACCESS_RO = 2'b01,
FIELD_ACCESS_RSVD = 2'b11
} field_access_e;
// my_reg_block_pkg.sv
package my_reg_block_pkg;
// 寄存器定义
class my_reg_block extends uvm_reg_block;
// 通道0控制寄存器
uvm_reg chnl0_ctrl;
// 通道1控制寄存器
uvm_reg chnl1_ctrl;
// 通道2控制寄存器
uvm_reg chnl2_ctrl;
// 通道0状态寄存器
uvm_reg chnl0_stat;
// 通道1状态寄存器
uvm_reg chnl1_stat;
// 通道2状态寄存器
uvm_reg chnl2_stat;
// 构造函数
function new(string name = "my_reg_block");
super.new(name, `UVM_NO_COVERAGE);
// 通道0控制寄存器
chnl0_ctrl = uvm_reg::type_id::create("chnl0_ctrl");
chnl0_ctrl.configure(this, null, REG_ADDR_CHNL0_CTRL, 32'h0, "RW", 32'h0, 0, 0);
chnl0_ctrl.set_access("RW");
// 通道1控制寄存器
chnl1_ctrl = uvm_reg::type_id::create("chnl1_ctrl");
chnl1_ctrl.configure(this, null, REG_ADDR_CHNL1_CTRL, 32'h0, "RW", 32'h0, 0, 0);
chnl1_ctrl.set_access("RW");
// 通道2控制寄存器
chnl2_ctrl = uvm_reg::type_id::create("chnl2_ctrl");
chnl2_ctrl.configure(this, null, REG_ADDR_CHNL2_CTRL, 32'h0, "RW", 32'h0, 0, 0);
chnl2_ctrl.set_access("RW");
// 通道0状态寄存器
chnl0_stat = uvm_reg::type_id::create("chnl0_stat");
chnl0_stat.configure(this, null, REG_ADDR_CHNL0_STAT, 32'h0, "RO", 32'h0, 7, 0);
chnl0_stat.set_access("RO");
// 通道1状态寄存器
chnl1_stat = uvm_reg::type_id::create("chnl1_stat");
chnl1_stat.configure(this, null, REG_ADDR_CHNL1_STAT, 32'h0, "RO", 32'h0, 7, 0);
chnl1_stat.set_access("RO");
// 通道2状态寄存器
chnl2_stat = uvm_reg::type_id::create("chnl2_stat");
chnl2_stat.configure(this, null, REG_ADDR_CHNL2_STAT, 32'h0, "RO", 32'h0, 7, 0);
chnl2_stat.set_access("RO");
endfunction
endclass
// 字段定义
class my_reg_field extends uvm_reg_field;
// 构造函数
function new(string name = "my_reg_field", int size = 1, int offset = 0, string access = "RW", bit reset = 0);
super.new(name, size, offset, access, reset, `UVM_NO_COVERAGE);
endfunction
endclass
// 通道0控制寄存器字段定义
class chnl0_ctrl_fields extends uvm_reg_fields;
// 通道使能
my_reg_field chnl_en;
// 优先级等级
my_reg_field prio_level;
// 数据包长度
my_reg_field pkt_len;
// 保留字段
my_reg_field reserved;
// 构造函数
function new(string name = "chnl0_ctrl_fields");
super.new(name, `UVM_NO_COVERAGE);
chnl_en = my_reg_field::type_id::create("chnl_en", 1, 0, "RW", 1'b0);
prio_level = my_reg_field::type_id::create("prio_level", 2, 1, "RW", 2'h0);
pkt_len = my_reg_field::type_id::create("pkt_len", 3, 3, "RW", 3'h0);
reserved = my_reg_field::type_id::create("reserved", 26, 6, "RO", 26'h0);
endfunction
endclass
// 通道1控制寄存器字段定义
class chnl1_ctrl_fields extends uvm_reg_fields;
// 通道使能
my_reg_field chnl_en;
// 优先级等级
my_reg_field prio_level;
// 数据包长度
my_reg_field pkt_len;
// 保留字段
my_reg_field reserved;
// 构造函数
function new(string name = "chnl1_ctrl_fields");
super.new(name, `UVM_NO_COVERAGE);
chnl_en = my_reg_field::type_id::create("chnl_en", 1, 0, "RW", 1'b0);
prio_level = my_reg_field::type_id::create("prio_level", 2, 1, "RW", 2'h0);
pkt_len = my_reg_field::type_id::create("pkt_len", 3, 3, "RW", 3'h0);
reserved = my_reg_field::type_id::create("reserved", 26, 6, "RO", 26'h0);
endfunction
endclass
// 通道2控制寄存器字段定义
class chnl2_ctrl_fields extends uvm_reg_fields;
// 通道使能
my_reg_field chnl_en;
// 优先级等级
my_reg_field prio_level;
// 数据包长度
my_reg_field pkt_len;
// 保留字段
my_reg_field reserved;
// 构造函数
function new(string name = "chnl2_ctrl_fields");
super.new(name, `UVM_NO_COVERAGE);
chnl_en = my_reg_field::type_id::create("chnl_en", 1, 0, "RW", 1'b0);
prio_level = my_reg_field::type_id::create("prio_level", 2, 1, "RW", 2'h0);
pkt_len = my_reg_field::type_id::create("pkt_len", 3, 3, "RW", 3'h0);
reserved = my_reg_field::type_id::create("reserved", 26, 6, "RO", 26'h0);
endfunction
endclass
// 通道0状态寄存器字段定义
class chnl0_stat_fields extends uvm_reg_fields;
// FIFO可用空间
my_reg_field fifo_avail;
// 保留字段
my_reg_field reserved;
// 构造函数
function new(string name = "chnl0_stat_fields");
super.new(name, `UVM_NO_COVERAGE);
fifo_avail = my_reg_field::type_id::create("fifo_avail", 8, 0, "RO", 8'h0);
reserved = my_reg_field::type_id::create("reserved", 24, 8, "RO", 24'h0);
endfunction
endclass
// 通道1状态寄存器字段定义
class chnl1_stat_fields extends uvm_reg_fields;
// FIFO可用空间
my_reg_field fifo_avail;
// 保留字段
my_reg_field reserved;
// 构造函数
function new(string name = "chnl1_stat_fields");
super.new(name, `UVM_NO_COVERAGE);
fifo_avail = my_reg_field::type_id::create("fifo_avail", 8, 0, "RO", 8'h0);
reserved = my_reg_field::type_id::create("reserved", 24, 8, "RO", 24'h0);
endfunction
endclass
// 通道2状态寄存器字段定义
class chnl2_stat_fields extends uvm_reg_fields;
// FIFO可用空间
my_reg_field fifo_avail;
// 保留字段
my_reg_field reserved;
// 构造函数
function new(string name = "chnl2_stat_fields");
super.new(name, `UVM_NO_COVERAGE);
fifo_avail = my_reg_field::type_id::create("fifo_avail", 8, 0, "RO", 8'h0);
reserved = my_reg_field::type_id::create("reserved", 24, 8, "RO", 24'h0);
endfunction
endclass
endpackage
```
以上代码中定义了一个名为my_reg_block_pkg的UVM RAL模型包,其中包含了一个名为my_reg_block的寄存器块,以及各个寄存器的字段定义。你可以根据自己的需要进行修改和扩展。
相关推荐
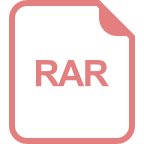
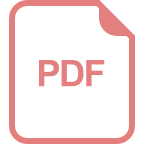














