英文字典生成,C语言
时间: 2023-09-30 16:09:41 浏览: 58
生成英文字典可以使用C语言的哈希表数据结构,以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LENGTH 50
#define TABLE_SIZE 1000
typedef struct {
char word[MAX_WORD_LENGTH];
char definition[MAX_WORD_LENGTH];
} Entry;
typedef struct {
Entry* entries[TABLE_SIZE];
} Dictionary;
int hash(char* word) {
int hash = 0;
for (int i = 0; i < strlen(word); i++) {
hash += word[i];
}
return hash % TABLE_SIZE;
}
void insert(Dictionary* dict, char* word, char* definition) {
Entry* entry = malloc(sizeof(Entry));
strcpy(entry->word, word);
strcpy(entry->definition, definition);
int index = hash(word);
dict->entries[index] = entry;
}
Entry* find(Dictionary* dict, char* word) {
int index = hash(word);
return dict->entries[index];
}
int main() {
Dictionary dict = {0};
insert(&dict, "apple", "a round fruit with red or green skin and a white inside");
insert(&dict, "banana", "a long curved fruit which grows in clusters and has soft pulpy flesh and yellow skin when ripe");
insert(&dict, "cherry", "a small, round, red fruit with a pit in the middle");
Entry* entry = find(&dict, "apple");
if (entry) {
printf("%s: %s\n", entry->word, entry->definition);
} else {
printf("Word not found\n");
}
return 0;
}
```
这个实现使用了一个简单的字符串哈希函数,将单词映射到哈希表中的一个位置。在插入时,将单词和定义保存在一个Entry结构体中,然后将其插入到哈希表中。在查找时,使用相同的哈希函数来确定位置,然后返回该位置上的Entry指针。如果该位置为空,则说明单词不存在。
相关推荐
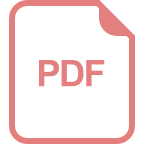
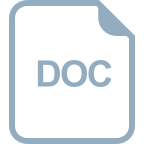
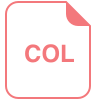
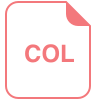
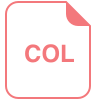
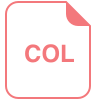
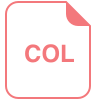









