c语言实现基于NTRU格的代理重加密技术代码
时间: 2024-01-23 20:02:52 浏览: 56
NTRU是一种非对称加密算法,可以用于代理重加密技术。以下是一个简单的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "ntru.h"
#define KEY_LEN 128
#define KEY_LEN_BITS 7
#define PLAIN_LEN 16
int main() {
uint8_t public_key[KEY_LEN];
uint8_t private_key[KEY_LEN];
ntru_gen_key_pair(public_key, private_key, KEY_LEN_BITS);
printf("Public key: ");
for (int i = 0; i < KEY_LEN; i++) {
printf("%02x", public_key[i]);
}
printf("\n");
printf("Private key: ");
for (int i = 0; i < KEY_LEN; i++) {
printf("%02x", private_key[i]);
}
printf("\n");
uint8_t plain_text[PLAIN_LEN] = "Hello, world!";
uint8_t cipher_text[KEY_LEN];
ntru_encrypt(cipher_text, public_key, KEY_LEN, plain_text, PLAIN_LEN);
printf("Cipher text: ");
for (int i = 0; i < KEY_LEN; i++) {
printf("%02x", cipher_text[i]);
}
printf("\n");
uint8_t decrypted_text[PLAIN_LEN];
ntru_decrypt(decrypted_text, private_key, KEY_LEN, cipher_text, KEY_LEN);
printf("Decrypted text: %s\n", decrypted_text);
return 0;
}
```
注意,这里的代码只是一个简单的示例,实际应用中需要考虑更多细节,例如密钥的安全存储和传输等。
阅读全文
相关推荐
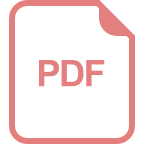








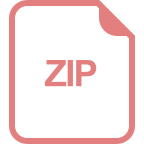
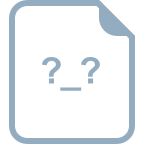
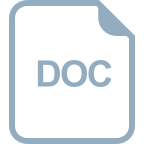



