请用c语言实现 (CBC或CFB或OFB之一) 的密文链模式算法 以上算法参见Stallings 网络安全与密码编码学(第6版)第3章、第5章和第6章。
时间: 2024-03-24 20:39:27 浏览: 89
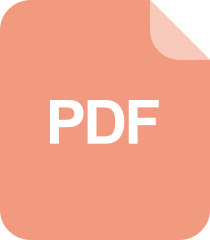
《密码编码学与网络安全》第四版课后详细答案.pdf

以下是使用CBC模式的AES加密和解密示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
#define AES_KEY_SIZE 128 // AES算法密钥长度
#define BLOCK_SIZE 16 // 分组大小
// 加密函数
void aes_encrypt(unsigned char *in, unsigned char *out, unsigned char *key, unsigned char *iv, int len){
AES_KEY aes_key;
// 设置加密密钥
AES_set_encrypt_key(key, AES_KEY_SIZE, &aes_key);
// 加密数据
unsigned char previous[BLOCK_SIZE];
memcpy(previous, iv, BLOCK_SIZE);
for (int i = 0; i < len; i += BLOCK_SIZE) {
for (int j = 0; j < BLOCK_SIZE; j++) {
out[i + j] = in[i + j] ^ previous[j];
}
AES_encrypt(out + i, out + i, &aes_key);
memcpy(previous, out + i, BLOCK_SIZE);
}
}
// 解密函数
void aes_decrypt(unsigned char *in, unsigned char *out, unsigned char *key, unsigned char *iv, int len){
AES_KEY aes_key;
// 设置解密密钥
AES_set_decrypt_key(key, AES_KEY_SIZE, &aes_key);
// 解密数据
unsigned char previous[BLOCK_SIZE];
memcpy(previous, iv, BLOCK_SIZE);
for (int i = 0; i < len; i += BLOCK_SIZE) {
AES_decrypt(in + i, out + i, &aes_key);
for (int j = 0; j < BLOCK_SIZE; j++) {
out[i + j] ^= previous[j];
}
memcpy(previous, in + i, BLOCK_SIZE);
}
}
int main(){
unsigned char key[AES_KEY_SIZE/8] = {0x00}; // 密钥
unsigned char iv[BLOCK_SIZE] = {0x00}; // 初始化向量
unsigned char in[] = "Hello World! This is a test message."; // 明文
unsigned char out[sizeof(in)]; // 密文
unsigned char decrypted[sizeof(in)]; // 解密后的明文
// 获取明文长度
int len = strlen((char *)in);
// 加密数据
aes_encrypt(in, out, key, iv, len);
// 输出加密结果
printf("Cipher text: ");
for (int i = 0; i < len; i++) {
printf("%02x", out[i]);
}
printf("\n");
// 解密数据
aes_decrypt(out, decrypted, key, iv, len);
// 输出解密结果
printf("Decrypted text: %s\n", decrypted);
return 0;
}
```
在这个示例中,我们使用了CBC模式的AES加密和解密算法。加密函数接受明文、密钥、初始化向量和数据长度作为输入,并输出密文。解密函数接受密文、密钥、初始化向量和数据长度作为输入,并输出解密后的明文。在加密和解密过程中,我们使用了一个previous数组来保存前一个分组的密文或明文,以便进行密文链的操作。
需要注意的是,我们使用了OpenSSL库中的AES函数来实现加密和解密。在实际应用中,需要根据具体需求选择合适的加密算法和模式,并根据具体情况对数据进行填充和对齐。
阅读全文
相关推荐
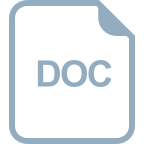
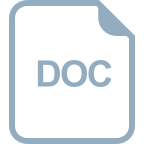
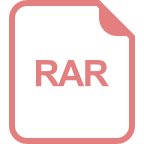
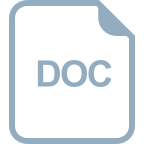

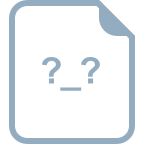
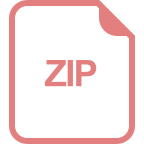
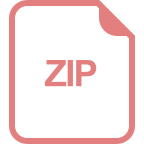
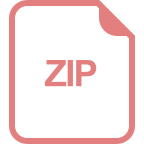
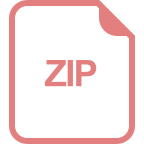
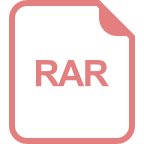
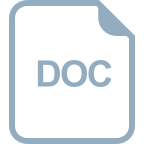
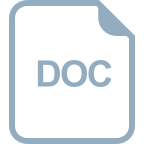
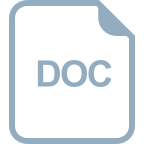




