写一个消除文法左递归的C语言程序,中间添加一个循环使程序可以反复输入,并在输入#时结束循环
时间: 2024-05-13 12:13:35 浏览: 215
的同时输出消除左递归后的文法。
消除左递归的C语言程序:
```
#include <stdio.h>
#include <string.h>
void eliminateLeftRecursion(char* nonTerminal, char productions[][10], int numProductions) {
char newNonTerminal[10];
int numNewProductions = 0;
char newProductions[numProductions][10];
int hasLeftRecursion = 0;
for (int i = 0; i < numProductions; i++) {
if (productions[i][0] == nonTerminal[0]) {
hasLeftRecursion = 1;
int len = strlen(productions[i]);
char alpha[len];
int alphaIndex = 0;
for (int j = 1; j < len; j++) {
if (productions[i][j] == '|') {
alpha[alphaIndex] = '\0';
alphaIndex = 0;
} else {
alpha[alphaIndex++] = productions[i][j];
}
}
alpha[alphaIndex] = '\0';
for (int j = 0; j < numProductions; j++) {
if (productions[j][0] == nonTerminal[0] && i != j) {
int len2 = strlen(productions[j]);
char beta[len2];
int betaIndex = 0;
for (int k = 1; k < len2; k++) {
if (productions[j][k] == '|') {
beta[betaIndex] = '\0';
betaIndex = 0;
} else {
beta[betaIndex++] = productions[j][k];
}
}
beta[betaIndex] = '\0';
newProductions[numNewProductions][0] = newNonTerminal[0];
int len3 = strlen(alpha);
for (int k = 0; k < len3; k++) {
newProductions[numNewProductions][k+1] = alpha[k];
}
int len4 = strlen(beta);
for (int k = 0; k < len4; k++) {
newProductions[numNewProductions+1][k+1] = beta[k];
}
newProductions[numNewProductions][len3+1] = newNonTerminal[1];
newProductions[numNewProductions+1][len4+1] = newNonTerminal[1];
numNewProductions += 2;
}
}
} else {
int len = strlen(productions[i]);
for (int j = 0; j < len; j++) {
newProductions[numNewProductions][j] = productions[i][j];
}
newProductions[numNewProductions++][len] = '\0';
}
}
if (hasLeftRecursion) {
printf("%c -> ", nonTerminal[0]);
for (int i = 0; i < numNewProductions-1; i++) {
printf("%s | ", newProductions[i]+1);
}
printf("%s\n", newProductions[numNewProductions-1]+1);
printf("%c' -> ", newNonTerminal[0]);
for (int i = 0; i < numNewProductions-1; i+=2) {
printf("%s%c' | ", newProductions[i]+1, newNonTerminal[1]);
}
printf("%s%c'\n", newProductions[numNewProductions-1]+1, newNonTerminal[1]);
} else {
printf("%c -> ", nonTerminal[0]);
for (int i = 0; i < numNewProductions-1; i++) {
printf("%s | ", newProductions[i]+1);
}
printf("%s\n", newProductions[numNewProductions-1]+1);
}
}
int main() {
while (1) {
int numProductions;
printf("请输入文法的产生式个数:");
scanf("%d", &numProductions);
char nonTerminal;
char productions[numProductions][10];
printf("请输入文法的每个产生式:\n");
for (int i = 0; i < numProductions; i++) {
scanf(" %c -> %[^\n]", &nonTerminal, productions[i]);
}
printf("消除左递归后的文法为:\n");
for (int i = 0; i < numProductions; i++) {
eliminateLeftRecursion(&nonTerminal, productions, numProductions);
}
printf("\n");
}
return 0;
}
```
程序中添加了一个无限循环,使得程序可以反复输入,并在输入的同时输出消除左递归后的文法。
阅读全文
相关推荐
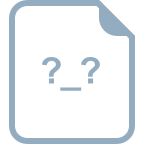

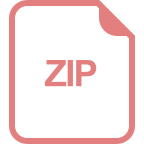
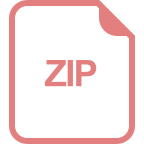
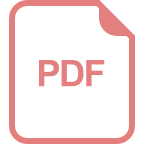
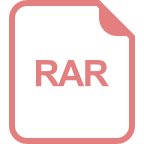
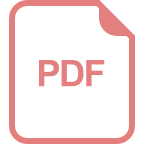
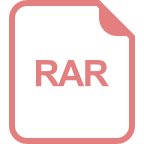
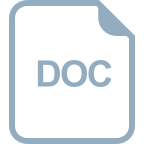
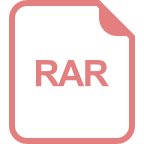
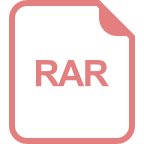
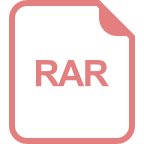
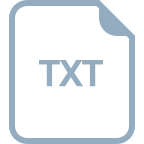
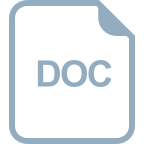
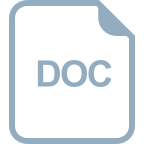
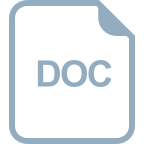
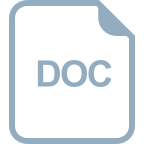