ptorch实现NSL-KDD数据集预处理
时间: 2024-04-29 22:23:46 浏览: 26
以下是使用ptorch实现NSL-KDD数据集预处理的步骤:
1. 下载NSL-KDD数据集,并将其解压缩。可以从以下链接下载数据集:http://www.unb.ca/cic/datasets/nsl.html
2. 使用Python的pandas库加载数据集,并将其转换为NumPy数组。例如,可以使用以下代码加载数据集:
```
import pandas as pd
import numpy as np
# Load the NSL-KDD dataset
train_df = pd.read_csv('KDDTrain+.txt', header=None)
test_df = pd.read_csv('KDDTest+.txt', header=None)
# Convert the datasets to NumPy arrays
train_data = train_df.to_numpy()
test_data = test_df.to_numpy()
```
3. 对数据进行预处理,包括对离散特征进行独热编码、对标签进行编码等。例如,可以使用以下代码进行预处理:
```
from sklearn.preprocessing import LabelEncoder, OneHotEncoder
# Encode the labels
label_encoder = LabelEncoder()
train_labels = label_encoder.fit_transform(train_data[:, -1])
test_labels = label_encoder.transform(test_data[:, -1])
# One-hot encode the categorical features
categorical_features = [1, 2, 3, 41]
one_hot_encoder = OneHotEncoder(categories='auto')
train_categorical = one_hot_encoder.fit_transform(train_data[:, categorical_features]).toarray()
test_categorical = one_hot_encoder.transform(test_data[:, categorical_features]).toarray()
# Scale the numerical features
numerical_features = [0, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40]
train_numerical = train_data[:, numerical_features].astype(np.float32)
test_numerical = test_data[:, numerical_features].astype(np.float32)
```
4. 将预处理后的数据合并为一个NumPy数组。例如,可以使用以下代码将处理后的数据合并起来:
```
# Combine the categorical and numerical features
train_features = np.concatenate((train_categorical, train_numerical), axis=1)
test_features = np.concatenate((test_categorical, test_numerical), axis=1)
# Combine the features and labels
train_data = np.concatenate((train_features, train_labels.reshape(-1, 1)), axis=1)
test_data = np.concatenate((test_features, test_labels.reshape(-1, 1)), axis=1)
```
5. 将处理后的数据保存到文件中,以便在训练和测试模型时使用。例如,可以使用以下代码将处理后的数据保存到文件中:
```
# Save the preprocessed data to files
np.save('train_data.npy', train_data)
np.save('test_data.npy', test_data)
```
这些步骤将NSL-KDD数据集进行预处理,使其可以在PyTorch中用于训练和测试模型。
相关推荐
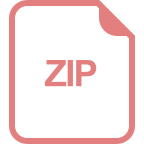











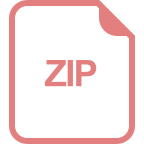
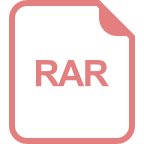