unity控制速度代码
时间: 2023-12-20 16:21:38 浏览: 32
Unity中控制物体速度的代码可以通过修改物体的刚体(Rigidbody)组件来实现。以下是一个简单的示例代码,可以在Update()函数中使用:
```csharp
public float speed = 10f; // 控制物体的速度
void Update() {
// 获取物体的刚体组件
Rigidbody rb = GetComponent<Rigidbody>();
// 计算移动方向
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontalInput, 0f, verticalInput);
// 设置物体速度
rb.velocity = movement * speed;
}
```
这个示例代码中,我们首先获取了物体的刚体组件,然后根据用户输入计算出物体的移动方向。最后,将移动方向乘以速度值,设置为物体的速度。这样就可以实现物体的移动了。
相关问题
unity 控制飞机代码
以下是一个基础的飞机控制代码示例,你可以在 Unity 中创建一个空对象,将此脚本附加到该对象上,并将飞机模型作为其子对象。然后您可以使用 WASD 或箭头键来控制飞机的移动,使用Q和E键或鼠标左右移动来控制飞机的旋转。
```csharp
using UnityEngine;
public class PlaneController : MonoBehaviour
{
public float speed = 10f; // 飞行速度
public float rotationSpeed = 100f; // 旋转速度
void Update()
{
// 控制飞机的移动
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
transform.Translate(Vector3.forward * Time.deltaTime * speed * vertical);
transform.Translate(Vector3.right * Time.deltaTime * speed * horizontal);
// 控制飞机的旋转
float rotation = Input.GetAxis("Rotation");
transform.Rotate(Vector3.up, Time.deltaTime * rotationSpeed * rotation);
}
}
```
请注意,这只是一个简单的示例,您可能需要根据自己的需求进行修改和调整。同时,这段代码也没有考虑到碰撞检测等其他方面,如果您需要实现更复杂的飞机控制逻辑,需要进一步扩展和完善。
unity角色控制代码怎么写
### 回答1:
Unity的角色控制代码可以通过使用Unity的脚本语言来编写,一般使用C#或者JavaScript,使用这些语言实现角色控制的基本流程是:1、创建角色控制器的实例;2、添加角色的控制脚本;3、实现角色控制的相关函数;4、测试和调试角色控制代码。
### 回答2:
Unity是一款流行的游戏开发引擎,其角色控制代码编写主要涉及以下几个方面。
首先,在Unity中创建一个角色模型并添加一个Capsule Collider组件作为角色的碰撞体。通过Rigidbody组件可以赋予角色物理属性,并使其受到物理引擎的控制。
接下来,编写角色控制脚本。可以创建一个C#脚本并将其附加到角色模型上。在脚本中,我们可以使用Input系统获取玩家的输入信息,例如键盘或鼠标输入。
通过Input系统获取到的输入信息包括角色的移动方向(比如前后左右)和角色的旋转方向。可以使用Transform组件的Translate方法来实现角色的移动,并使用Transform组件的Rotate方法来实现角色的旋转。
在移动角色时,可以使用Translate方法并传入移动方向向量乘以移动速度来控制角色的移动速度。比如,如果按下"W"键,则向量(0, 0, 1)表示向前移动;如果按下"S"键,则向量(0, 0, -1)表示向后移动。
在旋转角色时,可以使用Rotate方法并传入旋转方向向量乘以旋转速度来控制角色的旋转速度。比如,如果按下"A"键,则向量(0, -1, 0)表示向左旋转;如果按下"D"键,则向量(0, 1, 0)表示向右旋转。
此外,还可以添加一些额外的功能,如跳跃、奔跑、攻击等,可以根据游戏需求进行扩展。比如,可以通过添加一个Jump方法并使用Rigidbody组件的AddForce方法来实现角色的跳跃。
总而言之,Unity角色控制代码的编写就是通过获取玩家的输入信息,并根据输入信息来控制角色模型的移动和旋转,实现角色在游戏中的各种动作。
### 回答3:
Unity角色控制代码主要分为以下几个方面:
1. 获取角色输入:
通过Unity的输入系统(Input)来获取玩家对角色的输入。例如,使用Input.GetAxis("Horizontal")获取左右移动输入,Input.GetAxis("Vertical")获取前后移动输入,Input.GetButtonDown("Jump")获取跳跃输入等。
2. 控制角色移动:
使用刚体组件(Rigidbody)来控制角色的移动。通过获取到的输入,来修改角色的速度和方向。可以使用rigidbody.AddForce()方法来施加力来实现角色移动,也可以直接通过修改刚体的Velocity属性来实现。
3. 控制角色旋转:
通过修改角色的旋转来控制其朝向。可以使用Transform的Rotate()方法来实现,也可以使用刚体的angularVelocity属性直接修改角色的旋转速度。
4. 控制角色跳跃:
判断是否有跳跃输入,如果有则给角色添加一个向上的力以实现跳跃。可以使用rigidbody.AddForce()方法来施加向上的力,也可以使用刚体的Velocity属性来直接修改垂直速度。
5. 处理碰撞与重力:
使用碰撞器(Collider)来检测碰撞,并设置碰撞行为。例如,在碰到墙壁时停止向该方向的移动。同时,使用重力(通过刚体的useGravity属性来启用)来模拟角色受到的重力效果。
以上是对Unity角色控制代码的简要概述,根据具体需求和场景,还需要根据实际情况添加额外的逻辑、动画等来完善角色控制的效果。
相关推荐
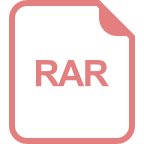
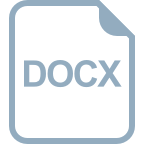
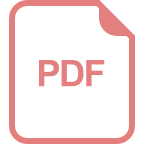
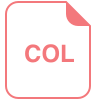
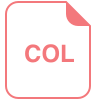
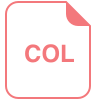
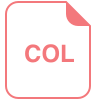
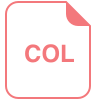







