用C++代码定义一个Cat类,其拥有静态数据成员numOfCats,记录已经创建的Cat对象的个体数目,静态数据成员numOfCatsGo,记录已经销毁的Cat对象的个体数目;静态成员函数getNumOfCats(bool IsConstruted),当IsConstruted为true时读取numOfCats,当IsConstruted为false时读取numOfCatsGo;数据私有成员weight,记录Cat对象的体重。 定义一个Boat类,其拥有数据私有成员weight,记录Boat对象的体重。 根据上述请完成如下内容: (1)请设计一个Cat类,体会静态数据成员和静态成员函数的用法。 (2)定义一个Cat类和Boat类的友元函数getTotalWeight(),计算一个Cat对象和Boat对象的体重和并返回。 输入格式 第一行输入正整数N,表示即将创建Cat类对象的个数, 第二行输入浮点数W0,表示一个Cat对象的体重 第三行输入浮点是W1,表示一个Boat对象的体重。 输出格式 首先先输出2N行数据,分别表示当前Cat对象创建时已经创建的对象个数,已经在内存中存在的对象个数;当前Cat对象销毁时,Cat对象在内存中存在的对象个数。(对应N个对象) 再输出1行表示Cat对象创建时已经创建的对象个数,已经在内存中存在的对象个数。(新创建一个对象,用于后续体重和计算) 然后输出一个Cat对象与一个Boat对象的体重和。 最后输出Cat对象销毁时,Cat对象在内存中存在的对象个数。 输入样例 复制 3 60.1 80.1 输出样例 复制 Cat Object is constructing, the constructed Number is 1, and the living Number is 1 Cat Object is deconstructing, and the living Number is 0 Cat Object is constructing, the constructed Number is 2, and the living Number is 1 Cat Object is deconstructing, and the living Number is 0 Cat Object is constructing, the constructed Number is 3, and the living Number is 1 Cat Object is deconstructing, and the living Number is 0 Cat Object is constructing, the constructed Number is 4, and the living Number is 1 140.2 Cat Object is deconstructing, and the living Number is 0
时间: 2024-02-23 16:01:11 浏览: 23
```cpp
#include <iostream>
using namespace std;
class Boat;
class Cat {
private:
static int numOfCats;
static int numOfCatsGo;
float weight;
public:
Cat(float w);
~Cat();
static int getNumOfCats(bool IsConstruted);
friend float getTotalWeight(Cat& c, Boat& b);
};
class Boat {
private:
float weight;
public:
Boat(float w) : weight(w) {}
friend float getTotalWeight(Cat& c, Boat& b);
};
int Cat::numOfCats = 0;
int Cat::numOfCatsGo = 0;
Cat::Cat(float w) : weight(w) {
numOfCats++;
cout << "Cat Object is constructing, the constructed Number is " << numOfCats << ", and the living Number is " << numOfCats - numOfCatsGo << endl;
}
Cat::~Cat() {
numOfCatsGo++;
cout << "Cat Object is deconstructing, and the living Number is " << numOfCats - numOfCatsGo << endl;
}
int Cat::getNumOfCats(bool IsConstruted) {
if (IsConstruted) {
return numOfCats;
} else {
return numOfCatsGo;
}
}
float getTotalWeight(Cat& c, Boat& b) {
return c.weight + b.weight;
}
int main() {
int N;
float w0, w1;
cin >> N >> w0 >> w1;
for (int i = 0; i < N; i++) {
Cat c(w0);
}
cout << "Cat Object is constructing, the constructed Number is " << Cat::getNumOfCats(true) << ", and the living Number is " << Cat::getNumOfCats(true) - Cat::getNumOfCats(false) << endl;
Boat b(w1);
cout << getTotalWeight(Cat(w0), b) << endl;
cout << "Cat Object is deconstructing, and the living Number is " << Cat::getNumOfCats(true) - Cat::getNumOfCats(false) << endl;
return 0;
}
```
相关推荐
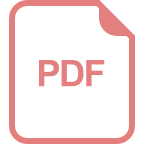
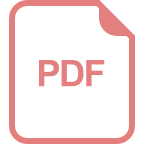
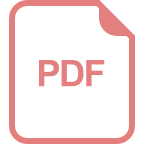













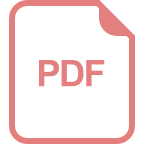