springboot2 和 oauth2 整合
时间: 2023-07-28 18:52:15 浏览: 79
Spring Boot 2 和 OAuth2 可以通过 Spring Security 实现集成。
下面是一个简单的 Spring Boot 2 OAuth2 集成的示例:
1. 在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.0.0.RELEASE</version>
</dependency>
```
2. 创建一个 WebSecurityConfigurerAdapter 类并重写 configure 方法:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/login").permitAll()
.antMatchers("/oauth/authorize").authenticated()
.and().formLogin().permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
}
```
3. 创建一个 ResourceServerConfigurerAdapter 类并重写 configure 方法:
```
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.and().authorizeRequests().anyRequest().permitAll();
}
}
```
4. 创建一个 AuthorizationServerConfigurerAdapter 类并重写 configure 方法:
```
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private UserDetailsService userDetailsService;
@Autowired
private DataSource dataSource;
@Bean
public TokenStore tokenStore() {
return new JdbcTokenStore(dataSource);
}
@Bean
public ClientDetailsService clientDetailsService() {
return new JdbcClientDetailsService(dataSource);
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.jdbc(dataSource);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenStore(tokenStore()).authenticationManager(authenticationManager).userDetailsService(userDetailsService);
}
}
```
5. 配置 application.yml 文件:
```
spring:
datasource:
url: jdbc:mysql://localhost:3306/oauth2
username: root
password: root
driver-class-name: com.mysql.jdbc.Driver
security:
oauth2:
client:
client-id: client
client-secret: secret
access-token-uri: http://localhost:8080/oauth/token
user-authorization-uri: http://localhost:8080/oauth/authorize
scope: read,write
resource:
user-info-uri: http://localhost:8080/api/userinfo
```
在上面的配置中,我们使用 MySQL 数据库存储 OAuth2 的数据。
6. 创建一个 UserController 类,用于测试:
```
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/userinfo")
public Principal user(Principal user) {
return user;
}
}
```
这个类提供了一个 /api/userinfo 接口,用于返回当前用户的信息。
7. 启动应用程序,并尝试访问 /api/userinfo 接口:
```
http://localhost:8080/api/userinfo
```
如果没有登录,将重定向到登录页面。登录后,您将获得当前用户的信息。
以上就是一个简单的 Spring Boot 2 OAuth2 集成的示例,可以根据自己的需求进行修改和扩展。
相关推荐
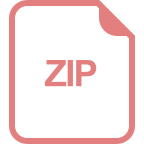
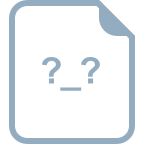













