springboot security整合oauth2
时间: 2023-09-19 10:09:56 浏览: 109
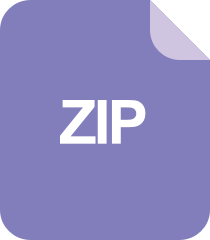
springboot整合oauth2
Spring Security是Spring框架的一个模块,提供了认证(Authentication)和授权(Authorization)的功能,而OAuth2是一种授权框架,它允许用户授权第三方应用访问其资源,而无需将用户名和密码提供给第三方应用。Spring Security可以与OAuth2进行整合,以实现安全的授权机制。
下面是Spring Boot与Spring Security整合OAuth2的步骤:
1.添加依赖
在pom.xml文件中添加Spring Security和OAuth2的依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
```
2.配置OAuth2认证服务器
在Spring Boot的配置文件application.yml中添加OAuth2认证服务器的配置信息,如下所示:
```
security:
oauth2:
client:
clientId: client_id
clientSecret: client_secret
accessTokenUri: http://localhost:8080/oauth/token
userAuthorizationUri: http://localhost:8080/oauth/authorize
resource:
userInfoUri: http://localhost:8080/user
```
其中,clientId和clientSecret是OAuth2客户端的标识和密码,accessTokenUri和userAuthorizationUri是OAuth2认证服务器的地址,userInfoUri是获取用户信息的地址。
3.配置Spring Security
在Spring Security的配置类中配置OAuth2的授权机制,如下所示:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private ClientDetailsService clientDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password("admin").roles("ADMIN")
.and()
.withUser("user").password("user").roles("USER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
@Bean
public TokenStore tokenStore() {
return new InMemoryTokenStore();
}
@Bean
@Autowired
public ApprovalStore approvalStore(TokenStore tokenStore) throws Exception {
TokenApprovalStore approvalStore = new TokenApprovalStore();
approvalStore.setTokenStore(tokenStore);
return approvalStore;
}
@Bean
@Autowired
public TokenServices tokenServices(ClientDetailsService clientDetailsService, TokenStore tokenStore) {
DefaultTokenServices tokenServices = new DefaultTokenServices();
tokenServices.setClientDetailsService(clientDetailsService);
tokenServices.setTokenStore(tokenStore);
tokenServices.setSupportRefreshToken(true);
return tokenServices;
}
@Bean
@Autowired
public OAuth2AuthenticationEntryPoint oAuth2AuthenticationEntryPoint(ResourceServerProperties sso) {
OAuth2AuthenticationEntryPoint entryPoint = new OAuth2AuthenticationEntryPoint();
entryPoint.setRealmName("spring-boot");
return entryPoint;
}
@Bean
@Autowired
public OAuth2AccessDeniedHandler oAuth2AccessDeniedHandler(ResourceServerProperties sso) {
OAuth2AccessDeniedHandler handler = new OAuth2AccessDeniedHandler();
return handler;
}
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring().antMatchers("/resources/**");
}
@Override
@Autowired
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService()).passwordEncoder(passwordEncoder());
}
@Override
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Bean
@Autowired
public ClientDetailsService clientDetailsService(DataSource dataSource) {
return new JdbcClientDetailsService(dataSource);
}
@Configuration
@EnableAuthorizationServer
protected static class OAuth2AuthorizationConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
private TokenStore tokenStore;
@Autowired
private ApprovalStore approvalStore;
@Autowired
private ClientDetailsService clientDetailsService;
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.withClientDetails(clientDetailsService);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenStore(tokenStore)
.approvalStore(approvalStore)
.authenticationManager(authenticationManager);
}
@Override
public void configure(AuthorizationServerSecurityConfigurer oauthServer) throws Exception {
oauthServer.realm("spring-boot");
}
}
}
```
其中,configure方法用于配置Spring Security的授权机制,tokenStore方法用于配置OAuth2的Token存储方式,approvalStore方法用于配置OAuth2的授权信息存储方式,tokenServices方法用于配置OAuth2的Token服务,oAuth2AuthenticationEntryPoint方法用于配置OAuth2的认证入口点,oAuth2AccessDeniedHandler方法用于配置OAuth2的拒绝访问处理器,authenticationManagerBean方法用于获取Spring Security的认证管理器,clientDetailsService方法用于配置OAuth2的客户端信息存储方式,OAuth2AuthorizationConfig类用于配置OAuth2的授权服务器。
4.测试
启动Spring Boot应用程序,打开浏览器,输入URL http://localhost:8080/login,进入登录页面,输入用户名和密码,点击登录按钮,进入首页,此时已经完成了OAuth2的授权机制。
阅读全文
相关推荐
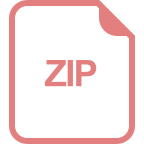


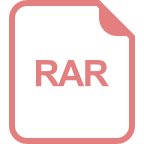
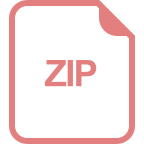
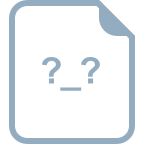
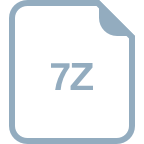







