cs5530 arduino
时间: 2023-07-29 12:11:22 浏览: 231
CS5530 is a precision analog-to-digital converter (ADC) chip designed by Cirrus Logic. It is not an Arduino board or a part of the Arduino platform. However, you can use an Arduino board to interface with the CS5530 ADC chip and read analog signals.
To interface with the CS5530 ADC chip, you will need to connect it to the SPI bus of the Arduino board. You will also need to write code to configure the CS5530 chip and read the data from it. The CS5530 datasheet and application notes provide detailed information on how to interface with the chip.
Here is an example code to read data from the CS5530 ADC chip using an Arduino board:
```c++
#include <SPI.h>
// CS5530 register addresses
#define CS5530_CTRL_REG 0x00
#define CS5530_DATA_REG 0x02
// CS5530 control register settings
#define CS5530_CTRL_PD 0x01
#define CS5530_CTRL_GAIN 0x02
void setup() {
Serial.begin(9600);
SPI.begin();
}
void loop() {
// Configure CS5530 chip
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE1));
digitalWrite(SS, LOW);
SPI.transfer(CS5530_CTRL_REG);
SPI.transfer(CS5530_CTRL_PD | CS5530_CTRL_GAIN); // Power up and set gain
digitalWrite(SS, HIGH);
SPI.endTransaction();
// Read data from CS5530 chip
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE1));
digitalWrite(SS, LOW);
SPI.transfer(CS5530_DATA_REG);
int data = (SPI.transfer(0) << 16) | (SPI.transfer(0) << 8) | SPI.transfer(0);
digitalWrite(SS, HIGH);
SPI.endTransaction();
Serial.print("CS5530 data: ");
Serial.println(data);
delay(1000);
}
```
Note that this is just an example code, and you may need to adjust it based on your specific requirements.
阅读全文
相关推荐
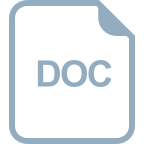

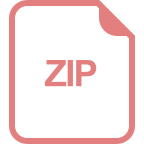
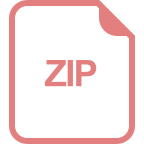
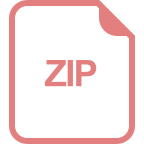
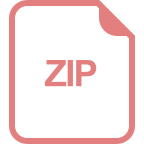
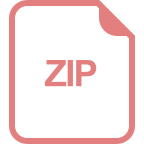
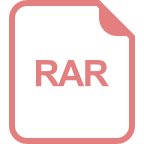
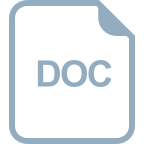
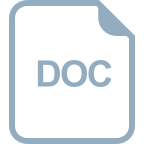




