C++ 编写程序,给定一组日期,按日期前后顺序排序。 输入 首先输入一个正整数n,表示n个日期 1<=n<=100。 此后n行,每行输入三个正整数,表示给定日期的年月日。 输出 排序之后的一组日期。
时间: 2023-12-03 08:47:11 浏览: 131
以下是使用 C++ 编写的程序,可以实现按日期前后顺序排序:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Date {
int year, month, day;
};
bool cmp(Date x, Date y) {
if (x.year != y.year) {
return x.year < y.year;
} else if (x.month != y.month) {
return x.month < y.month;
} else {
return x.day < y.day;
}
}
int main() {
int n;
cin >> n;
vector<Date> dates(n);
for (int i = 0; i < n; i++) {
cin >> dates[i].year >> dates[i].month >> dates[i].day;
}
sort(dates.begin(), dates.end(), cmp);
for (int i = 0; i < n; i++) {
cout << dates[i].year << " " << dates[i].month << " " << dates[i].day << endl;
}
return 0;
}
```
首先读入正整数 n,表示有 n 个日期。然后使用结构体 Date 存储每个日期的年月日,将它们存储在 vector 中。接着使用 sort 函数将 vector 中的日期按照年月日的顺序排序,最后依次输出排序后的日期即可。
需要注意的是,我们在排序时需要自定义一个比较函数 cmp,用于比较两个日期的大小。在 cmp 中,我们首先按年份升序排序,如果年份相同,则按月份升序排序,如果月份也相同,则按日期升序排序。
阅读全文
相关推荐
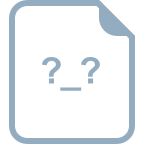
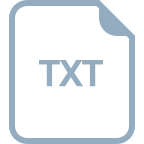

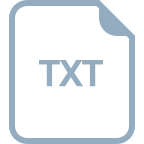
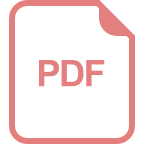
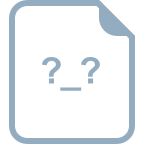
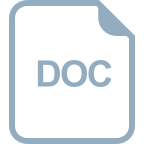
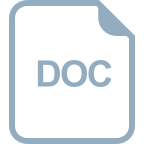
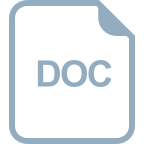
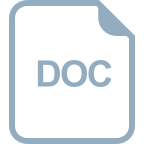
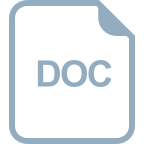
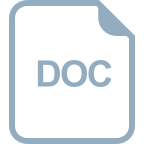
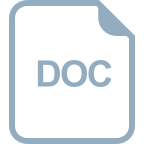
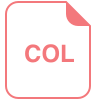
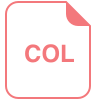
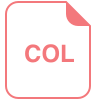
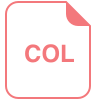