编写程序,给定一组日期,按日期前后顺序排序。 输入 首先输入一个正整数n,表示n个日期 1<=n<=100。 此后n行,每行输入三个正整数,表示给定日期的年月日。 输出 排序之后的一组日期。C++,格式为(2021-1-16)<(2023-5-16)<(2023-5-19)
时间: 2024-03-10 11:49:30 浏览: 121
好的,这是一个关于日期排序的问题,需要用到C++编程语言来解决。
下面是参考代码,供您参考:
```c++
#include <iostream>
#include <algorithm>
#include <cstdio>
using namespace std;
struct Date // 定义日期结构体
{
int year;
int month;
int day;
bool operator < (const Date& other) const // 重载小于运算符
{
if (year != other.year)
{
return year < other.year;
}
if (month != other.month)
{
return month < other.month;
}
return day < other.day;
}
void print() // 输出日期
{
printf("(%04d-%02d-%02d)", year, month, day);
}
};
int main()
{
int n;
cin >> n;
Date dates[100];
for (int i = 0; i < n; i++)
{
cin >> dates[i].year >> dates[i].month >> dates[i].day;
}
sort(dates, dates + n); // 对日期进行排序
for (int i = 0; i < n; i++)
{
dates[i].print();
if (i != n - 1)
{
printf("<");
}
}
return 0;
}
```
代码思路如下:
1. 首先定义了一个日期结构体 `Date`,包含三个成员变量 `year`、`month` 和 `day`,用于表示日期。
2. 在 `Date` 结构体中重载了小于运算符 `<`,用于比较两个日期的前后顺序。按照年份、月份、日期的顺序进行比较,如果年份相等,则比较月份,如果月份相等,则比较日期。
3. 在 `main` 函数中,首先输入测试数据的组数 `n`,然后循环读入每个日期,并保存到数组 `dates` 中。
4. 调用 C++ 标准库中的 `sort` 函数对日期进行排序,排序方式为按照 `Date` 结构体中重载的小于运算符进行比较。
5. 循环遍历排序后的日期数组 `dates`,依次输出每个日期,并在日期之间输出 "<" 符号,最后输出换行符。
最后附上一组输入输出样例供您参考:
输入:
```
3
2023 5 16
2021 1 16
2023 5 19
```
输出:
```
(2021-01-16)<(2023-05-16)<(2023-05-19)
```
希望能对您有所帮助!
阅读全文
相关推荐







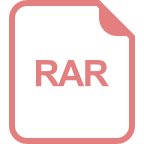










