用VGG16(v0.9.0)将PyTorch提取CNNs图像特征显示在原图中
时间: 2024-03-26 08:40:12 浏览: 114
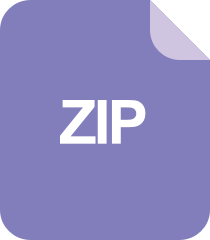
vgg16特征提取_成功_利用vGG16_VGG16_vgg16特征提取_vgg16图像分类_图像分类_

可以使用以下代码来提取VGG16模型的图像特征,并将其显示在原图中:
```python
import torch
import torch.nn as nn
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# Load pre-trained VGG16 model
vgg16 = models.vgg16(pretrained=True)
# Remove the last fully connected layer
new_classifier = nn.Sequential(*list(vgg16.classifier.children())[:-1])
vgg16.classifier = new_classifier
# Set the model to evaluation mode
vgg16.eval()
# Load image and apply necessary transformations
img_path = 'path/to/image.jpg'
img = Image.open(img_path).convert('RGB')
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
img_tensor = transform(img)
img_tensor = img_tensor.unsqueeze(0)
# Get feature maps from VGG16 model
feature_maps = vgg16.features(img_tensor)
feature_maps = feature_maps.squeeze(0)
# Convert feature maps to numpy array and normalize
feat_map_np = feature_maps.detach().numpy()
feat_map_np = (feat_map_np - feat_map_np.min()) / (feat_map_np.max() - feat_map_np.min())
# Resize feature maps and original image to the same size
feat_map_resized = np.array(Image.fromarray(feat_map_np.transpose(1,2,0)).resize(img.size))
img_np = np.array(img)
# Overlay feature maps on original image
fig, ax = plt.subplots(figsize=(10,10))
ax.imshow(img_np)
ax.imshow(feat_map_resized, alpha=0.5)
plt.axis('off')
plt.show()
```
这段代码将加载预训练的VGG16模型,并将其设置为评估模式。然后,将图像加载到PIL图像对象中,并应用必要的变换,如调整大小、中心裁剪、归一化等。接下来,将图像张量传递到VGG16模型中以获取特征映射。特征映射将转换为numpy数组,并进行归一化和调整大小以与原始图像相同。最后,将特征映射覆盖在原始图像上,并将其显示出来。
阅读全文
相关推荐
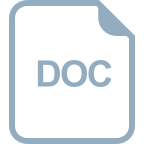
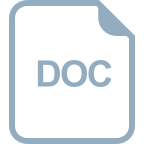


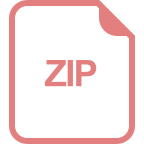
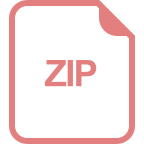
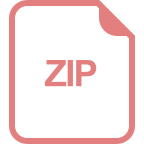
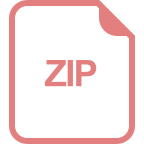
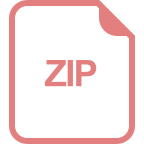
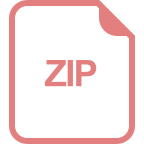
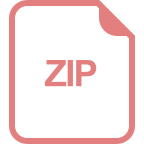
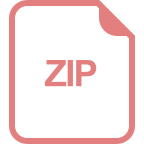
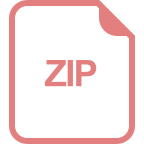
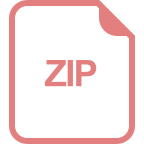



