function combination_count($n, $m) { $result = 1; for ($i = $n - $m + 1; $i <= $n; $i++) { $result *= $i; } for ($i = 1; $i <= $m; $i++) { $result /= $i; } return $result; } function get_combination_count($arr, $len) { $n = count($arr); $count = 0; for ($i = 0; $i < $len; $i++) { $count += combination_count($n - 1, $len - 1 - $i); $n --; } return $count; } $chars = array('a', 'D', '1', 't', 'R', 'I', 'f'); $len = 6; $count = get_combination_count($chars, $len);
时间: 2023-09-06 22:08:51 浏览: 154
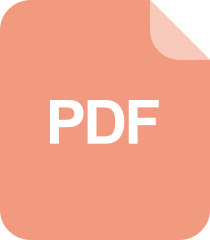
jQuery中(function($){})(jQuery)详解
根据您给出的代码,这段 PHP 代码定义了两个函数:`combination_count` 和 `get_combination_count`。
`combination_count` 函数用于计算组合数,输入两个参数 $n$ 和 $m$,返回 $C_n^m$ 的值。
`get_combination_count` 函数用于计算从给定字符集中取出指定长度的所有可能组合的数量,输入两个参数 $arr$ 和 $len$,其中 $arr$ 为字符集,$len$ 为组合的长度,返回从 $arr$ 中取出长度为 $len$ 的组合的总数。
代码最后给出一个示例,定义了字符集 `$chars` 和组合长度 `$len`,通过调用 `get_combination_count` 函数获取字符集 `$chars` 中长度为 `$len` 的所有组合的数量,结果保存在 `$count` 变量中。
阅读全文
相关推荐
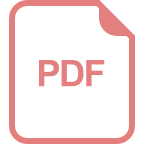
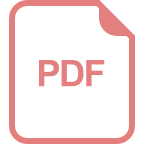
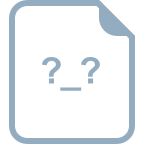
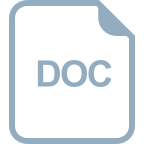
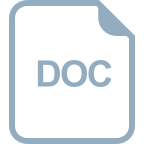
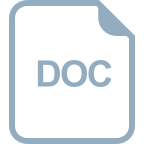
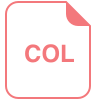
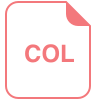



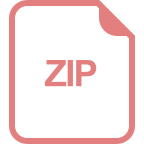
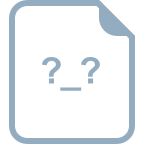
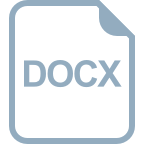
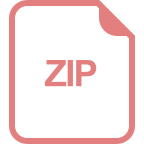
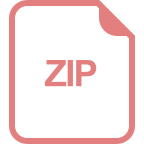