python实现Elgamal加密算法
时间: 2023-08-31 17:21:52 浏览: 115
### 回答1:
Elgamal加密算法是一种非对称加密算法,它基于离散对数问题。下面是Python实现Elgamal加密算法的示例代码:
```python
import random
from math import pow
a = random.randint(2, 10)
def gcd(a, b):
if a < b:
return gcd(b, a)
elif a % b == 0:
return b;
else:
return gcd(b, a % b)
# Generating large random numbers
def gen_key(q):
key = random.randint(pow(10, 20), q)
while gcd(q, key) != 1:
key = random.randint(pow(10, 20), q)
return key
# Modular exponentiation
def power(a, b, c):
x = 1
y = a
while b > 0:
if b % 2 == 0:
x = (x * y) % c
y = (y * y) % c
b = int(b / 2)
return x % c
# Asymmetric encryption
def encrypt(msg, q, h, g):
en_msg = []
k = gen_key(q) # Private key for sender
s = power(h, k, q)
p = power(g, k, q)
for i in range(0, len(msg)):
en_msg.append(msg[i])
print("g^k used : ", p)
print("g^ak used : ", s)
for i in range(0, len(en_msg)):
en_msg[i] = s * ord(en_msg[i])
return en_msg, p
def decrypt(en_msg, p, key, q):
dr_msg = []
h = power(p, key, q)
for i in range(0, len(en_msg)):
dr_msg.append(chr(int(en_msg[i] / h)))
return dr_msg
# Driver code
def main():
msg = 'encryption'
print("Original Message :", msg)
q = random.randint(pow(10, 20), pow(10, 50))
g = random.randint(2, q)
key = gen_key(q) # Private key for receiver
h = power(g, key, q)
print("g used : ", g)
print("g^a used : ", h)
en_msg, p = encrypt(msg, q, h, g)
dr_msg = decrypt(en_msg, p, key, q)
dmsg = ''.join(dr_msg)
print("Decrypted Message :", dmsg);
if __name__ == '__main__':
main()
```
这段代码中,我们使用了Python中的随机数模块(`random`)和数学模块(`math`),其中`random.randint(a,b)`函数用于生成a到b之间的随机整数,`pow(a,b,c)`函数用于计算a的b次方然后对c取模。在实现中,我们首先定义了一个随机数a,然后依次实现了生成密钥、模幂运算、加密和解密等功能。
在主函数`main()`中,我们定义了一个明文消息(`msg`),然后随机生成了一个素数q和一个整数g。接下来,我们分别生成了私钥和公钥,用公钥加密明文,再用私钥解密密文。最后输出了解密后的明文。
### 回答2:
Elgamal加密算法是一种非对称加密算法,用于保证数据的机密性。Python中可以通过以下步骤来实现Elgamal加密算法:
1. 选择两个大质数p和g,其中p是一个比要加密的数据大的素数,g是一个大于1且小于p-1的数。
2. 选择一个随机数a,使得1<a<p-1。
3. 计算g^a mod p的值,得到公钥h。
4. 将要加密的数据转换为整数形式。
5. 选择一个随机数k,使得1<k<p-1。
6. 计算g^k mod p的值,得到临时公钥。
7. 计算h^k mod p的值,得到临时私钥。
8. 将临时公钥、临时私钥和要加密的数据进行计算,得到密文。
9. 将密文发送给接收方。
10. 接收方使用自己的私钥a和密文进行计算,得到解密后的明文。
在Python中,可以通过大数运算库gmpy2来实现上述步骤。具体代码如下:
```
import gmpy2
def elgamal_encrypt(message, p, g, a):
h = gmpy2.powmod(g, a, p)
k = gmpy2.mpz_random()
temp_public_key = gmpy2.powmod(g, k, p)
temp_private_key = gmpy2.powmod(h, k, p)
ciphertext = (temp_public_key, (message * temp_private_key) % p)
return ciphertext
def elgamal_decrypt(ciphertext, p, a):
temp_public_key, encrypted_message = ciphertext
temp_private_key = gmpy2.powmod(temp_public_key, a, p)
plaintext = (encrypted_message * gmpy2.invert(temp_private_key, p)) % p
return plaintext
p = gmpy2.next_prime(gmpy2.mpz(2048))
g = gmpy2.mpz(2)
a = gmpy2.mpz_random()
message = 1234
ciphertext = elgamal_encrypt(message, p, g, a)
plaintext = elgamal_decrypt(ciphertext, p, a)
print("密文:", ciphertext)
print("明文:", plaintext)
```
上述代码使用了gmpy2库来进行大数的运算,通过调用`elgamal_encrypt`函数来加密数据,调用`elgamal_decrypt`函数来解密数据。最后打印出加密后的密文和解密后的明文。
需要注意的是,在实际应用中,还需要考虑到数据的安全性,如选择合适的素数p和随机数a,并进行适当的长度选择。
### 回答3:
Elgamal加密算法是一种非对称加密算法,常用于数据加密和安全通信领域。下面是一个使用Python实现Elgamal加密算法的示例代码:
```python
import random
# 生成大素数
def generate_prime_num():
prime_nums = [i for i in range(1000, 2000) if all(i % j != 0 for j in range(2, int(i ** 0.5) + 1))]
p = random.choice(prime_nums)
return p
# 生成本原元
def generate_primitive_root(p):
primitive_roots = []
for x in range(2, p):
if pow(x, p - 1, p) == 1:
primitive_roots.append(x)
g = random.choice(primitive_roots)
return g
# 密钥生成
def generate_keys():
p = generate_prime_num()
g = generate_primitive_root(p)
a = random.randint(2, p - 1)
h = pow(g, a, p)
public_key = (p, g, h)
private_key = a
return public_key, private_key
# 加密
def encrypt(plain_text, public_key):
p, g, h = public_key
r = random.randint(2, p - 1)
c1 = pow(g, r, p)
c2 = (plain_text * pow(h, r, p)) % p
cipher_text = (c1, c2)
return cipher_text
# 解密
def decrypt(cipher_text, private_key):
c1, c2 = cipher_text
p, _, _ = public_key
plain_text = (c2 * pow(c1, p - 1 - private_key, p)) % p
return plain_text
# 示例
public_key, private_key = generate_keys()
plain_text = 1234
cipher_text = encrypt(plain_text, public_key)
decrypted_text = decrypt(cipher_text, private_key)
print("公钥:", public_key)
print("私钥:", private_key)
print("明文:", plain_text)
print("加密后的密文:", cipher_text)
print("解密后的明文:", decrypted_text)
```
上面的代码实现了Elgamal加密算法的基本功能,包括密钥生成、加密和解密。可以根据实际需要对输入、输出进行适当修改。
阅读全文
相关推荐
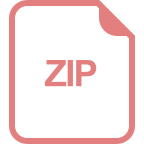
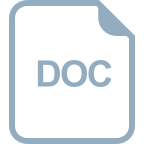
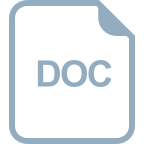
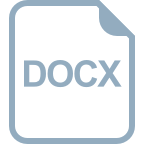
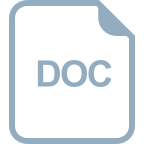
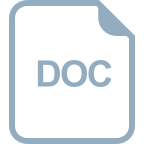
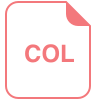
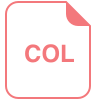







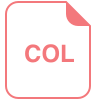