用java实现 编写GUI的双骰游戏。玩家掷两个骰子,计算两个骰子的点数之和。如果第一次掷的和是7或11,玩家赢;如果和为2、3或12则玩家输,庄家赢。如果和为其它数字,则成为玩家的“点数” ,继续掷骰子:如等于点数,玩家赢,如等于7,玩家输,否则,继续掷骰子。
时间: 2024-05-08 08:21:45 浏览: 17
以下是一个简单的 Java 实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
public class DiceGame extends JFrame implements ActionListener {
private final JButton rollButton;
private final JLabel resultLabel;
private int point = 0;
public DiceGame() {
super("Double Dice Game");
JPanel panel = new JPanel(new GridLayout(2, 1));
rollButton = new JButton("Roll the Dice");
rollButton.addActionListener(this);
panel.add(rollButton);
resultLabel = new JLabel("Click the button to roll the dice");
panel.add(resultLabel);
add(panel);
setSize(300, 150);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
int sum = rollDice();
if (point == 0) {
if (sum == 7 || sum == 11) {
resultLabel.setText("You win!");
} else if (sum == 2 || sum == 3 || sum == 12) {
resultLabel.setText("You lose!");
} else {
point = sum;
resultLabel.setText("Your point is " + point);
}
} else {
if (sum == point) {
resultLabel.setText("You win!");
point = 0;
} else if (sum == 7) {
resultLabel.setText("You lose!");
point = 0;
} else {
resultLabel.setText("Your point is " + point);
}
}
}
private int rollDice() {
Random random = new Random();
int dice1 = random.nextInt(6) + 1;
int dice2 = random.nextInt(6) + 1;
int sum = dice1 + dice2;
return sum;
}
public static void main(String[] args) {
new DiceGame();
}
}
```
在这个实现中,我们创建了一个 `JFrame` 窗口,并在其中放置了一个 `JPanel` 面板,上面有一个按钮和一个标签。当用户点击按钮时,我们将通过调用 `rollDice()` 方法来模拟掷骰子,并根据游戏规则来判断输赢。如果点数为7或11,则玩家胜利;如果点数为2、3或12,则玩家输;如果点数为其他数字,则这个数字成为玩家的点数,并且游戏将继续进行,直到玩家获胜或失败。当游戏结束时,我们将在标签中显示结果。
相关推荐
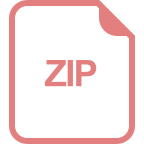
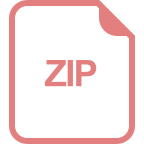














