java编写编写GUI的双骰游戏。玩家掷两个骰子,计算两个骰子的点数之和。如果第一次掷的和是7或11,玩家赢;如果和为2、3或12则玩家输,庄家赢。如果和为其它数字,则成为玩家的“点数” ,继续掷骰子:如等于点数,玩家赢,如等于7,玩家输,否则,继续掷骰子。
时间: 2024-05-08 18:21:43 浏览: 14
以下是一个简单的Java代码实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
public class DoubleDiceGame extends JFrame implements ActionListener {
private JButton btnRoll;
private JLabel lblResult;
private int point = 0;
private Random random = new Random();
public DoubleDiceGame() {
setTitle("Double Dice Game");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(2, 1));
btnRoll = new JButton("Roll");
btnRoll.addActionListener(this);
lblResult = new JLabel("Roll the dice!");
panel.add(btnRoll);
panel.add(lblResult);
add(panel);
setVisible(true);
}
public static void main(String[] args) {
new DoubleDiceGame();
}
@Override
public void actionPerformed(ActionEvent e) {
int sum = rollDice();
if (point == 0) {
if (sum == 7 || sum == 11) {
lblResult.setText("You win!");
} else if (sum == 2 || sum == 3 || sum == 12) {
lblResult.setText("You lose!");
} else {
point = sum;
lblResult.setText("Your point is " + point);
}
} else {
if (sum == point) {
lblResult.setText("You win!");
point = 0;
} else if (sum == 7) {
lblResult.setText("You lose!");
point = 0;
} else {
lblResult.setText("Roll again!");
}
}
}
private int rollDice() {
int dice1 = random.nextInt(6) + 1;
int dice2 = random.nextInt(6) + 1;
int sum = dice1 + dice2;
lblResult.setText("You rolled " + dice1 + " + " + dice2 + " = " + sum);
return sum;
}
}
```
这个程序使用了Swing库来创建GUI界面,并且实现了ActionListener接口来处理按钮点击事件。在程序中,rollDice()方法用来模拟掷骰子过程,计算两个骰子的点数之和并展示结果。在actionPerformed()方法中,根据点数的情况决定游戏的输赢,并更新界面上的提示信息。注意在每次游戏结束后需要将点数重置为0。
相关推荐
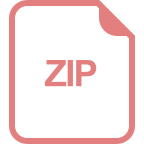
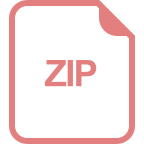














