判断TcpListener 端口是否被占用
时间: 2023-09-03 08:15:22 浏览: 156
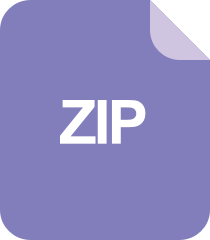
检测tcp端口是否被占用
在C#中,可以使用TcpListener类的TryCreate方法来判断指定端口是否被占用。这个方法会尝试创建一个TcpListener实例并绑定到指定端口,如果端口未被占用,则返回true,否则返回false。
以下是一个示例代码:
```
using System.Net;
using System.Net.Sockets;
public static bool IsPortInUse(int port)
{
bool inUse = false;
try
{
using (TcpClient tcpClient = new TcpClient())
{
tcpClient.Connect(IPAddress.Loopback, port);
inUse = true;
}
}
catch (SocketException)
{
// Port is unused and available.
}
return inUse;
}
```
这个方法会尝试连接到本地IP地址和指定端口的TcpListener,并捕获SocketException异常。如果连接成功,则说明端口已被占用,否则说明端口未被占用。
阅读全文
相关推荐
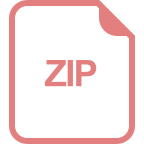
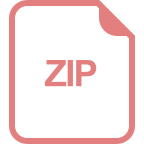
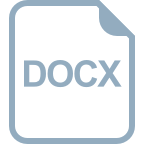
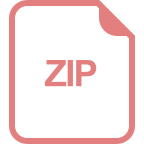
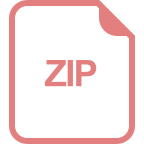