一个c语言程序完成顺序查找与折半查找输出元素位置、查找次数代码实现
时间: 2024-01-13 21:05:58 浏览: 30
以下是一个简单的C语言程序,用于实现顺序查找和折半查找,并输出元素位置和查找次数:
```c
#include <stdio.h>
int sequential_search(int arr[], int n, int x)
{
int i, count = 0;
for (i = 0; i < n; i++)
{
count++;
if (arr[i] == x)
return i;
}
printf("Total number of comparisons: %d\n", count);
return -1;
}
int binary_search(int arr[], int n, int x)
{
int low = 0, high = n - 1, mid, count = 0;
while (low <= high)
{
count++;
mid = (low + high) / 2;
if (arr[mid] == x)
{
printf("Total number of comparisons: %d\n", count);
return mid;
}
else if (arr[mid] < x)
low = mid + 1;
else
high = mid - 1;
}
printf("Total number of comparisons: %d\n", count);
return -1;
}
int main()
{
int arr[] = { 2, 5, 7, 10, 14, 16, 19, 20, 24, 28 };
int n = sizeof(arr) / sizeof(arr[0]);
int x = 16;
int pos = sequential_search(arr, n, x);
if (pos == -1)
printf("Element not found in the array.\n");
else
printf("Element found at position %d.\n", pos);
pos = binary_search(arr, n, x);
if (pos == -1)
printf("Element not found in the array.\n");
else
printf("Element found at position %d.\n", pos);
return 0;
}
```
在这个程序中,我们首先定义了两个函数,分别是顺序查找和折半查找。在顺序查找中,我们遍历整个数组,逐个比较每个元素,直到找到目标元素。在折半查找中,我们首先将数组分成两个部分,然后比较中间元素与目标元素的大小关系,如果中间元素等于目标元素,则返回中间元素的位置;否则,如果中间元素小于目标元素,则在右半部分进行查找;否则,在左半部分进行查找。
在主函数中,我们定义了一个整型数组和目标元素x。我们首先调用顺序查找函数,并输出元素位置(如果找到)或元素未找到。然后,我们调用折半查找函数,并再次输出元素位置(如果找到)或元素未找到。在两个函数中,我们还输出了查找次数。
注意:这只是一个简单的示例程序,实际上,顺序查找和折半查找的实现方式可能会有所不同,具体取决于应用场景和数据结构。
相关推荐
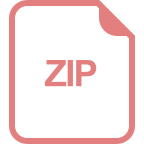
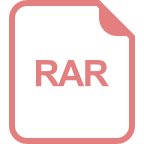
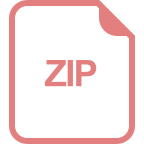














