已知一有序的序列1 3 5 7 9,采用顺序查找和折半查找法分别查找3和6(C语言)
时间: 2024-12-11 17:23:43 浏览: 7
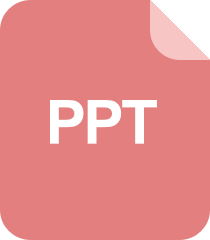
考研辅导--查找.ppt
在C语言中,我们可以分别编写顺序查找和折半查找的算法来在一个已排序的整数序列中查找特定值。
**顺序查找 (Linear Search)**:
这是一种最基础的查找算法,从数组的第一个元素开始逐个比较,直到找到目标值或遍历完整个数组。
```c
#include <stdio.h>
// 顺序查找函数
int sequential_search(int arr[], int target, int size) {
for (int i = 0; i < size; i++) {
if (arr[i] == target)
return i; // 找到返回位置
}
return -1; // 如果未找到,返回 -1 表示不存在
}
int main() {
int arr[] = {1, 3, 5, 7, 9};
int target = 3;
int size = sizeof(arr) / sizeof(arr[0]);
int index = sequential_search(arr, target, size);
if (index != -1)
printf("3的位置是 %d\n", index + 1); // +1 因为数组索引从0开始
else
printf("3在数组中未找到.\n");
return 0;
}
```
**折半查找 (Binary Search)**:
折半查找适用于已经排好序且大小固定的数组。它每次将搜索范围减半,通过比较中间元素和目标值来确定下一步搜索区间。
```c
#include <stdio.h>
#include <stdlib.h>
// 折半查找函数
int binary_search(int arr[], int target, int low, int high) {
while (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
low = mid + 1;
else
high = mid - 1;
}
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9};
int target = 6;
int size = sizeof(arr) / sizeof(arr[0]);
int index = binary_search(arr, target, 0, size - 1);
if (index != -1)
printf("6的位置是 %d\n", index + 1);
else
printf("6在数组中未找到.\n");
return 0;
}
```
阅读全文
相关推荐
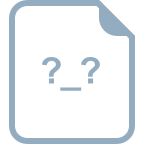
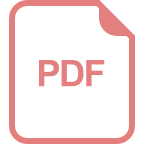















