php实现封装函数实现一个含有点线干扰元素的5位验证码,其中验证码包括英文大小写字母和数字,包含注释
时间: 2023-08-31 12:21:52 浏览: 65
### 回答1:
以下是一个用 PHP 实现的含有点线干扰元素的5位验证码的封装函数:
```php
/**
* 生成含有点线干扰元素的5位验证码
* 包括英文大小写字母和数字
*
* @return string 生成的验证码字符串
*/
function generateCaptcha() {
$captcha = ""; // 初始化验证码字符串
// 验证码字符集合
$charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
// 创建画布
$image = imagecreate(120, 40);
// 设置背景颜色(浅灰色)
$bg_color = imagecolorallocate($image, 220, 220, 220);
imagefill($image, 0, 0, $bg_color);
// 生成随机字符
for ($i = 0; $i < 5; $i++) {
$captcha .= $charset[mt_rand(0, strlen($charset) - 1)];
}
// 在画布上绘制字符
for ($i = 0; $i < 5; $i++) {
$text_color = imagecolorallocate($image, mt_rand(0, 150), mt_rand(0, 150), mt_rand(0, 150));
imagestring($image, 5, 20 * $i + 10, mt_rand(5, 20), $captcha[$i], $text_color);
}
// 绘制干扰点
for ($i = 0; $i < 50; $i++) {
$point_color = imagecolorallocate($image, mt_rand(0, 255), mt_rand(0, 255), mt_rand(0, 255));
imagesetpixel($image, mt_rand(0, 119), mt_rand(0, 39), $point_color);
}
// 绘制干扰线
for ($i = 0; $i < 5; $i++) {
$line_color = imagecolorallocate($image, mt_rand(0, 255), mt_rand(0, 255), mt_rand(0, 255));
imageline($image, mt_rand(0, 119), mt_rand(0, 39), mt_rand(0, 119), mt_rand(0, 39), $line_color);
}
// 输出图片并清除画布
header("Content-type: image/png");
imagepng($image);
imagedestroy($image);
return $captcha;
}
```
使用该函数可以生成一个含有点线干扰元素的5位验证码,并返回验证码字符串。该函数的实现过程如下:
1. 初始化验证码字符串 `$captcha`。
2. 定义验证码字符集合 `$charset`,包括英文大小写字母和数字。
3. 创建画布 `$image`,大小为 120x40 像素。
4. 设置画布背景颜色为浅灰色。
5. 生成随机字符,将其添加到验证码字符串 `$captcha` 中。
6. 在画布上绘制字符,使用随机颜色。
7. 在画布上绘制干扰点,随机位置、随机颜色。
8. 在画布上绘制干扰线,随机起点、随机终点、随机颜色。
9. 输出图片,并清除画布。
10. 返回验证码字符串 `$captcha`。
建议在使用该函数前先了解 PHP 中的图形处理函数,如 `imagecreate`、`imagecolorallocate`、`imagestring`、`imagesetpixel`、`imageline` 等。
### 回答2:
以下是一个用PHP实现封装函数生成含有点线干扰元素的5位验证码的示例代码:
```php
<?php
function generateCaptcha($width, $height, $length) {
// 创建一个新图像
$image = imagecreatetruecolor($width, $height);
// 定义颜色
$backgroundColor = imagecolorallocate($image, 255, 255, 255); // 背景颜色为白色
$textColor = imagecolorallocate($image, 0, 0, 0); // 文本颜色为黑色
$lineColor = imagecolorallocate($image, 200, 200, 200); // 干扰线颜色为灰色
$dotColor = imagecolorallocate($image, 100, 100, 100); // 干扰点颜色为深灰色
// 填充背景色
imagefill($image, 0, 0, $backgroundColor);
// 定义字母和数字字符集
$characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
// 生成随机验证码字符串
$captcha = '';
for ($i = 0; $i < $length; $i++) {
$captcha .= $characters[rand(0, strlen($characters) - 1)];
}
// 写入验证码文本
$font = 'path/to/font.ttf'; // 替换为实际字体文件路径
$textX = $width / ($length + 1);
$textY = $height / 1.5;
imagettftext($image, 30, 0, $textX, $textY, $textColor, $font, $captcha);
// 添加干扰线
for ($i = 0; $i < $width; $i += 5) {
imageline($image, $i, 0, $i, $height, $lineColor);
}
// 添加干扰点
for ($i = 0; $i < $width * $height / 4; $i++) {
imagesetpixel($image, rand(0, $width), rand(0, $height), $dotColor);
}
// 输出图像
header('Content-type: image/jpeg');
imagejpeg($image);
// 释放内存
imagedestroy($image);
// 返回验证码字符串
return $captcha;
}
// 使用示例
$captcha = generateCaptcha(200, 80, 5);
echo $captcha;
?>
```
以上示例代码中的`generateCaptcha`函数用于生成一个指定宽度、高度和长度的验证码。通过调用该函数,会生成一个包含英文大小写字母和数字的验证码,并在图像中添加了点线干扰元素。
### 回答3:
<?php
/**
* 生成一个含有点线干扰元素的5位验证码
* 验证码包括英文大小写字母和数字
*/
function generateCaptcha()
{
// 定义验证码字符集
$chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
// 创建一个画布
$image = imagecreate(120, 40);
// 设置画布背景色为白色
imagecolorallocate($image, 255, 255, 255);
// 生成干扰点
for ($i = 0; $i < 100; $i++) {
$x = rand(0, 120);
$y = rand(0, 40);
imagesetpixel($image, $x, $y, imagecolorallocate($image, rand(0, 255), rand(0, 255), rand(0, 255)));
}
// 生成干扰线
for ($i = 0; $i < 3; $i++) {
$x1 = rand(0, 120);
$y1 = rand(0, 40);
$x2 = rand(0, 120);
$y2 = rand(0, 40);
imageline($image, $x1, $y1, $x2, $y2, imagecolorallocate($image, rand(0, 255), rand(0, 255), rand(0, 255)));
}
// 生成验证码字符串
$code = '';
$length = strlen($chars);
for ($i = 0; $i < 5; $i++) {
$pos = rand(0, $length - 1);
$code .= $chars[$pos];
}
// 设置验证码文字颜色
$textColor = imagecolorallocate($image, rand(0, 255), rand(0, 255), rand(0, 255));
// 将验证码文字写入画布
imagettftext($image, 20, 0, 30, 30, $textColor, 'path/to/font.ttf', $code);
// 输出图像并释放内存
header('Content-Type: image/png');
imagepng($image);
imagedestroy($image);
return $code;
}
// 使用示例
$captcha = generateCaptcha();
echo $captcha;
?>
以上代码是一个使用PHP实现的生成含有点线干扰元素的5位验证码的函数。首先,我们定义了一个包含英文大小写字母和数字的字符集。然后,我们创建了一个画布并设置画布的背景色为白色。接下来,我们使用循环生成100个随机位置的干扰点,并为每个干扰点随机设置颜色。然后,我们使用循环生成3条随机位置和颜色的干扰线。接下来,我们使用循环随机生成5位验证码的字符串。然后,我们设置验证码文字的颜色,并使用imagettftext函数将验证码文字写入画布。最后,我们输出图像,并返回生成的验证码字符串。
相关推荐
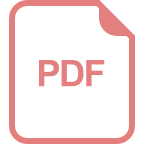
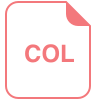













