用python栈写一个迷宫问题
时间: 2024-01-04 22:03:49 浏览: 35
以下是一个基本的迷宫问题解决方案,使用深度优先搜索和栈来遍历迷宫。请注意,此代码假定迷宫是一个二维数组,其中0表示可通过的通道,1表示墙壁。此外,代码还假定迷宫的起点为(0,0),终点为(n-1,n-1)(其中n是迷宫的大小)。
```python
# 定义一个栈类
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
# 定义一个迷宫类
class Maze:
def __init__(self, maze):
self.maze = maze
self.n = len(maze)
# 检查当前位置是否可走
def is_valid_move(self, x, y):
if x < 0 or x >= self.n or y < 0 or y >= self.n:
return False
elif self.maze[x][y] == 1:
return False
else:
return True
# 搜索迷宫
def solve(self):
stack = Stack()
visited = set()
stack.push((0, 0))
while not stack.is_empty():
x, y = stack.pop()
if (x, y) in visited:
continue
visited.add((x, y))
if x == self.n - 1 and y == self.n - 1:
return True
if self.is_valid_move(x + 1, y):
stack.push((x + 1, y))
if self.is_valid_move(x - 1, y):
stack.push((x - 1, y))
if self.is_valid_move(x, y + 1):
stack.push((x, y + 1))
if self.is_valid_move(x, y - 1):
stack.push((x, y - 1))
return False
# 测试代码
maze = [
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[1, 0, 1, 1, 0],
[1, 0, 0, 1, 0],
[1, 1, 0, 0, 0]
]
m = Maze(maze)
print(m.solve()) # 输出True
```
以上代码使用深度优先搜索和栈来遍历迷宫,并在找到终点时返回True。如果无法到达终点,则返回False。
相关推荐
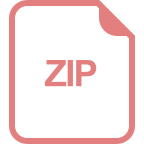
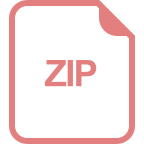
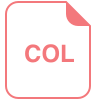
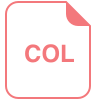
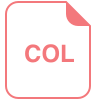
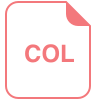
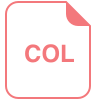









