如何用python求时滞微分方程数值解
时间: 2024-03-21 19:13:21 浏览: 23
要用Python求解时滞微分方程的数值解,可以使用scipy.integrate库中的delayed函数。具体步骤如下:
1. 导入必要的库:
```python
import numpy as np
from scipy.integrate import solve_delayed
```
2. 定义时滞微分方程:
```python
def f(t, y, yd):
tau = 1.0
dydt = -y + yd(t - tau)
return dydt
```
其中,t表示时间,y表示未知函数,yd表示时滞函数,tau表示时间延迟。
3. 定义初始条件和时间网格:
```python
y0 = [1.0]
tmax = 10.0
dt = 0.01
tgrid = np.arange(0, tmax+dt, dt)
```
其中,y0表示y在t=0时的值,tmax表示模拟的最长时间,dt表示时间步长,tgrid表示时间网格。
4. 定义时滞函数:
```python
def yd(u):
return np.interp(u, tgrid, yhist)
```
其中,u表示时滞时间,yhist表示y在之前时间的值。
5. 解方程:
```python
sol = solve_delayed(f, y0, tgrid, yd)
```
其中,sol是一个对象,包含了数值解和其他信息。
6. 提取数值解:
```python
y = sol.y[0]
```
其中,sol.y是一个数组,包含了数值解。
完整代码如下:
```python
import numpy as np
from scipy.integrate import solve_delayed
def f(t, y, yd):
tau = 1.0
dydt = -y + yd(t - tau)
return dydt
y0 = [1.0]
tmax = 10.0
dt = 0.01
tgrid = np.arange(0, tmax+dt, dt)
def yd(u):
return np.interp(u, tgrid, yhist)
yhist = np.zeros_like(tgrid)
yhist[0] = y0[0]
for i in range(1, len(tgrid)):
yhist[i] = yhist[i-1] + dt * (-yhist[i-1])
sol = solve_delayed(f, y0, tgrid, yd)
y = sol.y[0]
import matplotlib.pyplot as plt
plt.plot(tgrid, y, label='y')
plt.legend()
plt.show()
```
这个例子中,时滞函数是y在之前时间的值,因此我们先用欧拉法求出y在所有时间点的值,然后用np.interp函数将其转换为时滞函数。最后,我们求解时滞微分方程并绘制数值解。
相关推荐
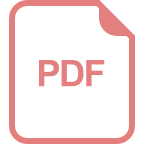














