用C语言,统计成绩:从键盘输入10个学生的学号、姓名,以及数学、语文、英语成绩,写到文本文件f3.txt中,再从文件中取出数据,计算每个学生的总成绩和平均分,并将结果显示在屏幕上
时间: 2023-06-27 16:03:32 浏览: 136
以下是用C语言统计成绩并将结果写入文件的代码:
```c
#include <stdio.h>
typedef struct {
int id;
char name[20];
int math;
int chinese;
int english;
} Student;
int main() {
FILE *fp;
fp = fopen("f3.txt", "w"); // 打开文件,以写入模式
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
Student student[10];
for (int i = 0; i < 10; i++) {
printf("请输入第%d个学生的学号、姓名、数学成绩、语文成绩和英语成绩: ", i + 1);
scanf("%d %s %d %d %d", &student[i].id, student[i].name, &student[i].math, &student[i].chinese, &student[i].english);
fprintf(fp, "%d %s %d %d %d\n", student[i].id, student[i].name, student[i].math, student[i].chinese, student[i].english); // 将数据写入文件
}
fclose(fp); // 关闭文件
fp = fopen("f3.txt", "r"); // 打开文件,以读取模式
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
printf("学号\t姓名\t数学成绩\t语文成绩\t英语成绩\t总成绩\t平均分\n");
for (int i = 0; i < 10; i++) {
fscanf(fp, "%d %s %d %d %d", &student[i].id, student[i].name, &student[i].math, &student[i].chinese, &student[i].english); // 从文件中读取数据
int total = student[i].math + student[i].chinese + student[i].english;
float average = (float) total / 3;
printf("%d\t%s\t%d\t\t%d\t\t%d\t\t%d\t%.2f\n", student[i].id, student[i].name, student[i].math, student[i].chinese, student[i].english, total, average); // 输出结果
}
fclose(fp); // 关闭文件
return 0;
}
```
以上代码可以实现统计成绩并将结果写入文件,再从文件中读取数据计算每个学生的总成绩和平均分,并将结果显示在屏幕上。
阅读全文
相关推荐
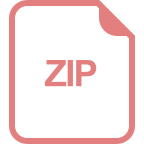
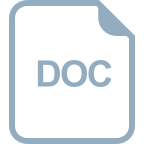















