朴素贝叶斯代码实现,Java
时间: 2024-01-02 14:41:54 浏览: 87
很高兴为您解答,以下是Java语言实现朴素贝叶斯算法的代码示例:
首先,定义一个类 NaiveBayesClassifier:
```
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class NaiveBayesClassifier {
private Map<String, Integer> labelMap; // 每个标签对应的文本数目
private Map<String, Integer> featureCountMap; // 每个特征出现的总次数
private Map<String, Map<String, Integer>> featureLabelCountMap; // 每个特征在每个标签下的次数
private int totalCount = 0; // 总文本数目
public NaiveBayesClassifier() {
labelMap = new HashMap<>();
featureCountMap = new HashMap<>();
featureLabelCountMap = new HashMap<>();
}
// 添加一条文本及其对应的标签
public void addText(String text, String label) {
String[] words = text.split(" ");
for (String word : words) {
totalCount++;
featureCountMap.put(word, featureCountMap.getOrDefault(word, 0) + 1); // 特征出现次数加1
if (!labelMap.containsKey(label)) {
labelMap.put(label, 1);
featureLabelCountMap.put(label, new HashMap<>());
} else {
labelMap.put(label, labelMap.get(label) + 1);
}
Map<String, Integer> featureCount = featureLabelCountMap.get(label);
featureCount.put(word, featureCount.getOrDefault(word, 0) + 1); // 特征在该标签下的次数加1
}
}
// 给定一条文本,预测其标签
public String predict(String text) {
String[] words = text.split(" ");
Map<String, Double> labelScoreMap = new HashMap<>(); // 每个标签的得分
for (String label : labelMap.keySet()) {
double labelScore = Math.log((double) labelMap.get(label) / totalCount); // 计算标签的先验概率
for (String word : words) {
double featureCount = featureLabelCountMap.get(label).getOrDefault(word, 0);
labelScore += Math.log((featureCount + 1) / (labelMap.get(label) + featureCountMap.size())); // 计算每个特征在该标签下的条件概率
}
labelScoreMap.put(label, labelScore);
}
double maxScore = Double.NEGATIVE_INFINITY;
String maxLabel = null;
for (String label : labelScoreMap.keySet()) {
double labelScore = labelScoreMap.get(label);
if (labelScore > maxScore) {
maxScore = labelScore;
maxLabel = label;
}
}
return maxLabel;
}
}
```
其中,labelMap表示每个标签对应的文本数目,featureCountMap表示每个特征出现的总次数,featureLabelCountMap表示每个特征在每个标签下的次数。在addText()方法中,我们将每个文本及其对应的标签加入到classifier中。在predict()方法中,我们计算每个标签的概率分数,然后返回分数最高的标签。
下面是一个使用示例:
```
public static void main(String[] args) {
List<String> texts = new ArrayList<>();
texts.add("Chinese Beijing Chinese");
texts.add("Chinese Chinese Shanghai");
texts.add("Chinese Macao");
texts.add("Tokyo Japan Chinese");
NaiveBayesClassifier classifier = new NaiveBayesClassifier();
for (String text : texts) {
String[] parts = text.split(" ");
String label = parts[parts.length - 1];
String content = text.substring(0, text.lastIndexOf(" "));
classifier.addText(content, label);
}
String text = "Chinese Chinese Chinese Tokyo Japan";
String label = classifier.predict(text);
System.out.println(label);
}
```
通过这段代码,我们可以将四条文本分成两类("Chinese"和"Tokyo"),并且成功预测了一条新的文本的标签。这就是朴素贝叶斯算法的基本思想。
阅读全文
相关推荐
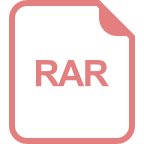
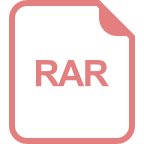

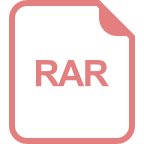
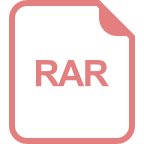
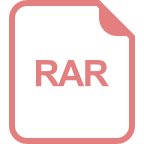
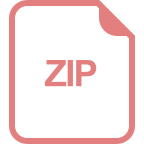
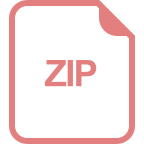
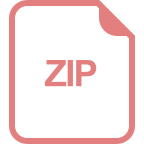
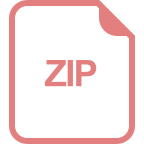
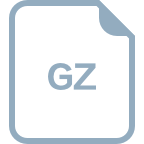
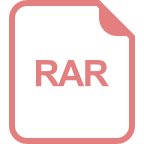
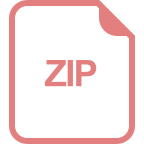
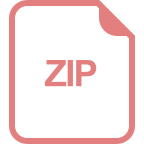
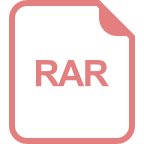

