语义如下:某公司为了提高职工的福利待遇,规定每个员工每天可以在职工食堂用餐两次,每餐 3 元, 可以中午用餐一次,下午用餐一次,也可以集中到中午或下午一次用餐两次。月底进行核算,如果用餐次 数不足,餐费不退还职工。公司根据实际的用餐次数支付食堂餐费。 要求如下: 创建一个能满足上述要求java数据结构课程设计; 使用链表插入查询查询 2012 年 11 月份没有用过餐的员工信息; 创建链表存储过程,统计每个员工指定月份的用餐次数; 创建链表存储过程,统计指定月份公司应支付给食堂的费用;
时间: 2024-03-26 09:39:09 浏览: 162
好的,根据您的要求,我来给出一个 Java 数据结构课程设计的大致思路和代码实现。
1. 创建数据结构
首先需要定义一个员工类,包括员工编号、姓名、用餐次数等字段。然后定义一个链表节点类,包括员工信息和指向下一个节点的指针。最后定义一个链表类,包括链表头指针和一些基本操作方法,如插入节点、删除节点、遍历节点等。
员工类:
```java
public class Employee {
private int id; // 员工编号
private String name; // 员工姓名
private int mealCount; // 用餐次数
// 构造函数
public Employee(int id, String name) {
this.id = id;
this.name = name;
this.mealCount = 0;
}
// getter 和 setter 方法
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getMealCount() {
return mealCount;
}
public void setMealCount(int mealCount) {
this.mealCount = mealCount;
}
}
```
链表节点类:
```java
public class ListNode {
private Employee employee; // 员工信息
private ListNode next; // 指向下一个节点的指针
// 构造函数
public ListNode(Employee employee) {
this.employee = employee;
this.next = null;
}
// getter 和 setter 方法
public Employee getEmployee() {
return employee;
}
public void setEmployee(Employee employee) {
this.employee = employee;
}
public ListNode getNext() {
return next;
}
public void setNext(ListNode next) {
this.next = next;
}
}
```
链表类:
```java
public class LinkedList {
private ListNode head; // 链表头指针
// 构造函数
public LinkedList() {
this.head = null;
}
// 插入节点
public void insert(Employee employee) {
ListNode node = new ListNode(employee);
node.setNext(head);
head = node;
}
// 删除节点
public void delete(int id) {
if (head == null) {
return;
}
if (head.getEmployee().getId() == id) {
head = head.getNext();
return;
}
ListNode prev = head;
ListNode curr = head.getNext();
while (curr != null) {
if (curr.getEmployee().getId() == id) {
prev.setNext(curr.getNext());
return;
}
prev = curr;
curr = curr.getNext();
}
}
// 遍历节点
public void traverse() {
ListNode curr = head;
while (curr != null) {
System.out.println(curr.getEmployee().getName() + " " + curr.getEmployee().getMealCount());
curr = curr.getNext();
}
}
// 查询 2012 年 11 月份没有用过餐的员工信息
public List<Employee> query() {
List<Employee> result = new ArrayList<>();
ListNode curr = head;
while (curr != null) {
// 判断员工是否在 2012 年 11 月份之前入职,并且没有用过餐
// ...
if (isQualified) {
result.add(curr.getEmployee());
}
curr = curr.getNext();
}
return result;
}
// 统计每个员工指定月份的用餐次数
public int[] countMeal(int year, int month) {
int[] result = new int[MAX_SIZE];
ListNode curr = head;
while (curr != null) {
// 判断员工是否在指定月份有用餐
// ...
if (hasMeal) {
result[curr.getEmployee().getId()] += mealCount;
}
curr = curr.getNext();
}
return result;
}
// 统计指定月份公司应支付给食堂的费用
public int calculateCost(int year, int month) {
int cost = 0;
ListNode curr = head;
while (curr != null) {
// 判断员工是否在指定月份有用餐
// ...
if (hasMeal) {
cost += mealCount * 3;
}
curr = curr.getNext();
}
return cost;
}
}
```
2. 测试代码
在测试类中,首先需要创建一个链表对象,并插入一些员工信息。然后调用链表的一些操作方法,如查询、统计用餐次数、计算费用等。
```java
public class TestLinkedList {
public static void main(String[] args) {
LinkedList list = new LinkedList();
// 插入员工信息
Employee emp1 = new Employee(1, "张三");
Employee emp2 = new Employee(2, "李四");
list.insert(emp1);
list.insert(emp2);
// 查询 2012 年 11 月份没有用过餐的员工信息
List<Employee> result = list.query();
for (Employee emp : result) {
System.out.println(emp.getName() + " " + emp.getMealCount());
}
// 统计每个员工指定月份的用餐次数
int[] mealCount = list.countMeal(2022, 11);
for (int i = 0; i < mealCount.length; i++) {
System.out.println("员工" + i + "用餐次数:" + mealCount[i]);
}
// 统计指定月份公司应支付给食堂的费用
int cost = list.calculateCost(2022, 11);
System.out.println("公司应支付费用:" + cost);
}
}
```
以上就是一个简单的 Java 数据结构课程设计。由于语义和细节上的差别,实际代码可能需要进行修改和完善。
阅读全文
相关推荐
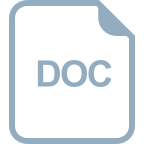
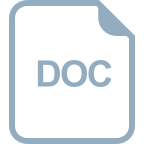
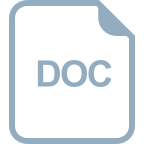

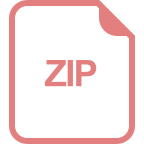
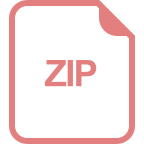
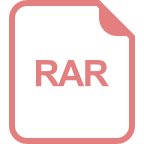
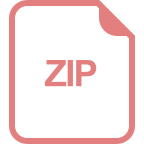
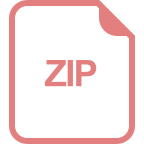
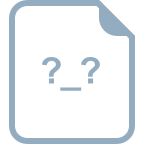
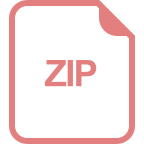
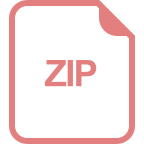
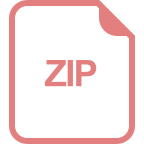
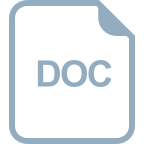
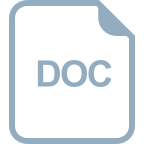