c#实现实时监控一个文件夹中是否产生新的txt文件,如果产生新的txt文件,就判断这个文件是不是可编辑,如果可编辑,就在这个文件原有内容的头部添加信息,并将修改后的txt文件转化为csv格式,保存到另一个文件夹中,转化过程显示在控制台上
时间: 2024-05-16 09:13:04 浏览: 18
你可以使用FileSystemWatcher类来实现文件夹中是否产生新文件的监控,并使用StreamReader类来读取文件内容,判断文件是否可编辑,使用StreamWriter类来向文件头部添加信息,并使用TextFieldParser类来将txt文件转化为csv格式并保存到另一个文件夹中。以下是代码示例:
```csharp
using System;
using System.IO;
using Microsoft.VisualBasic.FileIO;
class Program
{
static void Main(string[] args)
{
// 监控文件夹
FileSystemWatcher watcher = new FileSystemWatcher();
watcher.Path = @"C:\test"; // 文件夹路径
watcher.Filter = "*.txt"; // 监控txt文件
watcher.EnableRaisingEvents = true;
watcher.Created += new FileSystemEventHandler(OnCreated);
Console.WriteLine("正在监控文件夹 {0} 中是否产生新的txt文件...", watcher.Path);
Console.ReadLine();
}
static void OnCreated(object source, FileSystemEventArgs e)
{
if (File.Exists(e.FullPath))
{
// 判断文件是否可编辑
try
{
using (FileStream fs = File.OpenWrite(e.FullPath))
{
// 文件可编辑,添加信息到头部
using (StreamWriter sw = new StreamWriter(fs))
{
sw.WriteLine("添加的信息");
sw.Flush();
}
}
// 转化为csv格式并保存到另一个文件夹中
string fileName = Path.GetFileNameWithoutExtension(e.FullPath) + ".csv";
string sourcePath = e.FullPath;
string targetPath = @"C:\csv"; // 保存csv文件的文件夹路径
string targetFile = Path.Combine(targetPath, fileName);
using (TextFieldParser parser = new TextFieldParser(sourcePath))
{
parser.TextFieldType = FieldType.Delimited;
parser.SetDelimiters(",");
using (StreamWriter sw = new StreamWriter(targetFile))
{
while (!parser.EndOfData)
{
string[] fields = parser.ReadFields();
for (int i = 0; i < fields.Length; i++)
{
sw.Write(fields[i]);
if (i < fields.Length - 1)
{
sw.Write(",");
}
}
sw.WriteLine();
}
sw.Flush();
}
}
Console.WriteLine("文件 {0} 已添加信息并转化为csv格式保存到文件夹 {1} 中。", e.Name, targetPath);
}
catch (UnauthorizedAccessException)
{
// 文件不可编辑,忽略
}
catch (Exception ex)
{
Console.WriteLine("处理文件 {0} 时发生错误: {1}", e.Name, ex.Message);
}
}
}
}
```
相关推荐
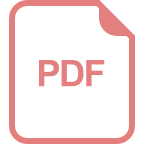














