iterator获取map键
时间: 2023-09-06 20:14:12 浏览: 72
可以使用C++中的迭代器来遍历map的键。以下是一个例子:
```c++
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "one";
myMap[2] = "two";
myMap[3] = "three";
// 遍历map的键
std::map<int, std::string>::iterator it;
for (it = myMap.begin(); it != myMap.end(); it++) {
std::cout << it->first << std::endl;
}
return 0;
}
```
在上面的例子中,我们定义了一个`std::map<int, std::string>`类型的变量`myMap`,并将一些键值对添加到其中。然后,我们使用迭代器`it`遍历map的键,即`it->first`。最后,我们输出了每个键的值。
相关问题
Iterator遍历map
Iterator遍历map的步骤如下:
1. 首先,通过调用map.entrySet()方法,将map转换为一个包含键值对的Set集合。
2. 然后,通过调用集合的iterator()方法获取一个Iterator对象。
3. 接下来,使用while循环和Iterator的hasNext()方法来判断是否还有下一个元素。
4. 在循环中,通过调用Iterator的next()方法来获取下一个键值对,并将其保存在一个Entry对象中。
5. 最后,可以通过Entry对象的getKey()和getValue()方法来分别获取键和值。
至于为什么Entry的首字母要大写,这是因为Entry是一个接口,按照Java命名规范,接口的名称应该以大写字母开头。
QMapIterator遍历map
你可以使用QMapIterator来遍历QMap。以下是示例代码:
```c++
QMap<QString, int> map;
map.insert("One", 1);
map.insert("Two", 2);
map.insert("Three", 3);
QMapIterator<QString, int> i(map);
while (i.hasNext()) {
i.next();
qDebug() << i.key() << ": " << i.value();
}
```
上述代码将输出以下结果:
```
One: 1
Three: 3
Two: 2
```
在上述代码中,我们首先创建了一个QMap对象并向其中插入了一些键值对。然后,我们使用QMapIterator来遍历map,并使用i.key()和i.value()获取当前项的键和值。最后,我们使用qDebug()输出了这些键值对。
阅读全文
相关推荐
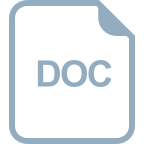
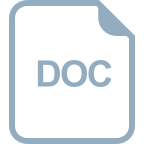
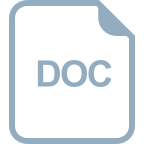


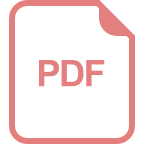








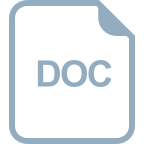
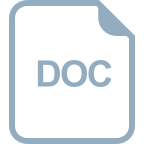
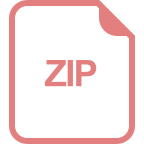