怎么遍历map的key
时间: 2023-10-13 12:20:35 浏览: 32
可以使用迭代器遍历map的key,示例如下:
```c++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = {{"apple", 2}, {"banana", 3}, {"orange", 4}};
// 使用迭代器遍历map的key
std::map<std::string, int>::iterator iter;
for (iter = myMap.begin(); iter != myMap.end(); iter++) {
std::cout << iter->first << std::endl;
}
return 0;
}
```
输出结果为:
```
apple
banana
orange
```
也可以使用auto关键字简化代码,示例如下:
```c++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = {{"apple", 2}, {"banana", 3}, {"orange", 4}};
// 使用auto关键字遍历map的key
for (auto iter = myMap.begin(); iter != myMap.end(); iter++) {
std::cout << iter->first << std::endl;
}
return 0;
}
```
输出结果同样为:
```
apple
banana
orange
```
注意,以上示例代码中,我们只输出了map的key,如果需要输出value,只需要将 `iter->first` 改为 `iter->second` 即可。
相关推荐
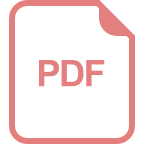
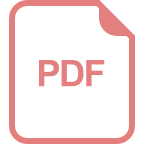














