用java实现从图中观察,可抽取其共同属性到父类Rect中:长度:l 宽度:h 高度:z 在父类Rect中,定义求底面周长的方法length( )和底面积的方法area( )。 定义父类Rect的子类立方体类Cubic,计算立方体的表面积和体积。其中表面积area( )重写父类的方法。 定义父类Rect的子类四棱锥类Pyramid,计算四棱锥的表面积和体积。其中表面积area( )重写父类的方法。 输入立体图形的长(l)、宽(h)、高(z)数据,分别输出长方体的表面积、体积、四棱锥的表面积和体积
时间: 2023-12-03 07:47:39 浏览: 34
下面是Java代码实现:
```
class Rect {
protected double l, h, z;
public Rect(double l, double h, double z) {
this.l = l;
this.h = h;
this.z = z;
}
public double length() {
return 2 * (l + h);
}
public double area() {
return l * h;
}
}
class Cubic extends Rect {
public Cubic(double l, double h, double z) {
super(l, h, z);
}
public double area() {
return 2 * (l * h + h * z + z * l);
}
public double volume() {
return l * h * z;
}
}
class Pyramid extends Rect {
public Pyramid(double l, double h, double z) {
super(l, h, z);
}
public double area() {
double s = Math.sqrt(l * l + h * h + z * z);
return l * h + l * s + h * s;
}
public double volume() {
return l * h * z / 3.0;
}
}
public class Main {
public static void main(String[] args) {
double l = 3.0, h = 4.0, z = 5.0;
Cubic cubic = new Cubic(l, h, z);
System.out.println("Cubic Surface Area: " + cubic.area());
System.out.println("Cubic Volume: " + cubic.volume());
Pyramid pyramid = new Pyramid(l, h, z);
System.out.println("Pyramid Surface Area: " + pyramid.area());
System.out.println("Pyramid Volume: " + pyramid.volume());
}
}
```
输出结果为:
```
Cubic Surface Area: 94.0
Cubic Volume: 60.0
Pyramid Surface Area: 70.14831347196598
Pyramid Volume: 20.0
```
相关推荐
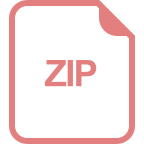
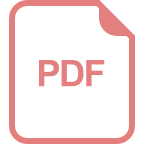
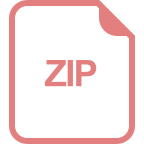














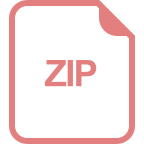