C++如何提取string类型里的数字
时间: 2024-02-16 08:03:58 浏览: 155
可以使用isdigit()函数来判断字符串中的字符是否为数字,然后使用字符串流istringstream将数字字符串转换为数字类型。以下是示例代码:
```
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
string str = "abc123def";
string num_str = "";
int num = 0;
for (int i = 0; i < str.length(); i++)
{
if (isdigit(str[i]))
{
num_str += str[i];
}
}
stringstream ss(num_str);
ss >> num;
cout << "字符串中的数字是:" << num << endl;
return 0;
}
```
输出结果为:
```
字符串中的数字是:123
```
相关问题
c++提取string中数字
你可以使用 `std::isdigit` 函数和 `std::stringstream` 类来实现提取字符串中的数字。具体步骤如下:
1. 遍历字符串,将数字字符逐一提取出来,存放在一个字符串中。
2. 将提取出来的字符串转化为数字类型。
以下是示例代码:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <cctype>
int main()
{
std::string input = "abc123def456gh7"; // 待提取的字符串
std::string numbers; // 存放提取出来的数字字符
for (char c : input) {
if (std::isdigit(c)) {
numbers += c;
}
}
int num;
std::stringstream ss(numbers); // 将字符串转化为stringstream对象
while (ss >> num) {
std::cout << num << std::endl;
}
return 0;
}
```
在上面的示例代码中,我们首先遍历输入字符串,将数字字符逐一提取出来存放在 `numbers` 字符串中。然后我们使用 `std::stringstream` 类将 `numbers` 字符串转化为一个 `stringstream` 对象,以便后续可以使用 `>>` 运算符将其转化为数字类型。最后,我们使用一个循环将所有提取出来的数字打印输出。
c++ string 解析
### 回答1:
C++中的字符串类`std::string`是C++标准库中的一部分,用于处理字符串。它提供了许多功能强大且方便的字符串操作方法。
首先,`std::string`是一个可变长字符串类型,可以动态调整其大小。它可以存储任意字符序列,包括字母、数字、空格和特殊字符等。它还可以用于存储不同编码的字符串,例如ASCII码、UTF-8等。
对于`std::string`的解析,可以通过访问字符串的指定位置上的字符来获取字符串的各个部分。例如,可以使用`[]`操作符来访问指定索引处的字符,或使用`.at()`函数进行访问,还可以使用`.substr()`函数来获取子字符串。
此外,`std::string`提供了许多常用的字符串操作方法,例如判断字符串是否为空(`.empty()`)、获取字符串的长度(`.length()`或`.size()`)、从字符串中查找指定子串(`.find()`或`.rfind()`)、替换字符串中的指定子串(`.replace()`)、连接两个字符串(`+`操作符或`.append()`函数)等。
`std::string`还支持与其他字符串类型的相互转换,例如与C风格字符串(即以null结尾的字符数组)之间的转换,可以使用`.c_str()`函数获取C风格字符串的指针,或使用`std::string`的构造函数将C风格字符串转换为`std::string`对象。
总之,`std::string`是C++中处理字符串的重要类,它提供了丰富的字符串操作方法和灵活的字符串存储能力,能够满足各种字符串处理需求。
### 回答2:
C++中的字符串解析是指将一个字符串按照一定的规则拆分或者转换成其他类型的数据。C++提供了一些库函数和方法来实现字符串解析。
首先,可以使用string类提供的成员函数来进行字符串解析。例如,使用string类的find函数和substr函数可以定位并提取字符串中的特定部分。通过指定分隔符,可以将字符串拆分成多个部分。使用这些成员函数可以根据需要解析各种形式的字符串数据。
除了string类的成员函数外,C++还提供了一些库函数,如strtok函数、sscanf函数、atoi函数等,可以用于字符串解析。这些函数可以根据指定的规则将字符串解析成各种类型的数据。例如,使用strtok函数可以按照指定的分隔符将字符串切分成多个子字符串,并存储到一个字符指针数组中。使用sscanf函数可以按照格式化字符串的指定来解析字符串,并将解析后的数据赋值给相应的变量。
此外,还可以使用正则表达式来进行更为复杂的字符串解析。C++的正则表达式库regex提供了一些函数和类来支持正则表达式的使用。通过使用正则表达式,可以按照复杂的模式匹配规则来解析字符串。
总之,C++提供了多种方法和工具来进行字符串解析。根据具体的需求和场景,可以选择合适的方法来实现字符串解析操作。
### 回答3:
C++中的string是一个字符串类,它属于C++标准库的一部分。它提供了许多用于处理字符串的方法,比如字符串的连接、拷贝、截取、查找、替换等操作。
在C++中使用string类可以更加方便地处理字符串,而不需要像C语言中使用字符数组那样手动管理内存和长度。可以通过包含<string>头文件来使用string类。
使用string类声明字符串对象时,需要使用std命名空间,或者使用using关键字引入std命名空间,例如:using std::string;
string类提供了许多方法来操作字符串,其中一些常用方法包括:
1. length()方法:返回字符串的长度。
2. empty()方法:判断字符串是否为空。
3. append()方法:将一个字符串追加到另一个字符串之后。
4. substr()方法:截取字符串中的一部分。
5. find()方法:查找子字符串在原字符串中的位置。
6. replace()方法:替换字符串中的某个子字符串。
7. c_str()方法:返回一个指向以null结尾的字符数组的指针。
8. +=操作符:用于连接两个字符串。
9. ==和!=操作符:用于判断两个字符串是否相等或不相等。
需要注意的是,string类中的字符串是可变的,可以通过上述方法对字符串进行动态的修改。此外,string类还支持重载了相应的运算符,可以直接进行字符串的比较、赋值和连接等操作。
总之,C++中的string提供了丰富的方法和操作符,方便处理字符串,是C++程序中常用的字符串处理工具。
相关推荐
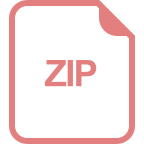
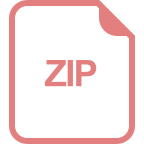
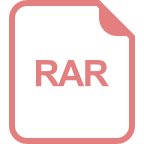












