在tf的环境下 搭建resnet18_attention,训练cifa10,打印出运行结果 使用了数据增强和学习率衰减等技术,为了尽可能提高准确率。
时间: 2024-04-30 20:24:32 浏览: 11
好的,以下是搭建ResNet18_Attention并训练CIFAR-10的代码及解释:
首先,你需要导入必要的库和模块:
``` python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers, models, regularizers
from tensorflow.keras.preprocessing.image import ImageDataGenerator
```
接着,你需要定义ResNet18_Attention模型:
``` python
def resnet18_attention(input_shape, num_classes):
input = layers.Input(shape=input_shape)
# conv1
x = layers.Conv2D(filters=64, kernel_size=(7,7), strides=(2,2), padding='same', kernel_regularizer=regularizers.l2(1e-4))(input)
x = layers.BatchNormalization()(x)
x = layers.Activation('relu')(x)
x = layers.MaxPooling2D(pool_size=(3,3), strides=(2,2), padding='same')(x)
# conv2_x
x = resnet_block(x, filters=64, blocks=2, strides=(1,1))
x = attention_block(x)
# conv3_x
x = resnet_block(x, filters=128, blocks=2, strides=(2,2))
x = attention_block(x)
# conv4_x
x = resnet_block(x, filters=256, blocks=2, strides=(2,2))
x = attention_block(x)
# conv5_x
x = resnet_block(x, filters=512, blocks=2, strides=(2,2))
x = attention_block(x)
x = layers.GlobalAveragePooling2D()(x)
output = layers.Dense(num_classes, activation='softmax')(x)
model = models.Model(inputs=input, outputs=output)
return model
```
这个ResNet18_Attention模型是由ResNet18和注意力机制所组成的,其中ResNet18由4个stage组成,每个stage中包含多个残差块,而注意力机制则是用来提取图像中不同区域的重要性,从而提高分类的准确性。
ResNet18中的残差块由两个卷积层和一个恒等映射组成,其中第一个卷积层的卷积核大小为3x3,第二个卷积层的卷积核大小也为3x3,但是它的卷积核数目是第一个卷积层的两倍。恒等映射用来保证x和F(x)的维度相同。每个stage的第一个残差块的第一个卷积层的步长为2,以便在输入和输出之间建立空间尺寸的降采样。
注意力机制由两个全连接层和一个Sigmoid激活函数组成,用来计算图像中每个区域的重要性,从而提高分类的准确性。
接着,你需要定义ResNet18_Attention的残差块:
``` python
def resnet_block(input_tensor, filters, blocks, strides):
x = input_tensor
for i in range(blocks):
strides = (strides, strides) if i == 0 else (1, 1)
x = resnet_identity_block(x, filters=filters, strides=strides)
return x
def resnet_identity_block(input_tensor, filters, strides):
x = layers.Conv2D(filters=filters, kernel_size=(3,3), strides=strides, padding='same', kernel_regularizer=regularizers.l2(1e-4))(input_tensor)
x = layers.BatchNormalization()(x)
x = layers.Activation('relu')(x)
x = layers.Conv2D(filters=filters, kernel_size=(3,3), strides=(1,1), padding='same', kernel_regularizer=regularizers.l2(1e-4))(x)
x = layers.BatchNormalization()(x)
x = layers.add([x, input_tensor])
x = layers.Activation('relu')(x)
return x
```
这里定义的残差块是由两个卷积层和一个恒等映射组成的,其中第一个卷积层的卷积核大小为3x3,第二个卷积层的卷积核大小也为3x3,但是它的卷积核数目是第一个卷积层的两倍。恒等映射用来保证x和F(x)的维度相同。
接着,你需要定义ResNet18_Attention的注意力机制:
``` python
def attention_block(input_tensor):
x = layers.GlobalAveragePooling2D()(input_tensor)
x = layers.Dense(units=int(x.shape[-1]), activation='relu')(x)
x = layers.Dense(units=int(x.shape[-1]), activation='sigmoid')(x)
x = tf.reshape(x, [-1, 1, 1, int(x.shape[-1])])
x = input_tensor * x
return x
```
这里定义的注意力机制由两个全连接层和一个Sigmoid激活函数组成,用来计算图像中每个区域的重要性,从而提高分类的准确性。
接下来,你需要对CIFAR-10数据集进行数据增强和准备:
``` python
(train_images, train_labels), (test_images, test_labels) = keras.datasets.cifar10.load_data()
train_images = train_images.astype('float32') / 255.0
test_images = test_images.astype('float32') / 255.0
train_labels = keras.utils.to_categorical(train_labels, 10)
test_labels = keras.utils.to_categorical(test_labels, 10)
data_generator = ImageDataGenerator(rotation_range=15,
width_shift_range=0.1,
height_shift_range=0.1,
horizontal_flip=True,
vertical_flip=False)
data_generator.fit(train_images)
```
这里使用了Keras中的CIFAR-10数据集,并对其进行了归一化处理。数据增强包括旋转、平移和翻转等,可以增加数据集的数量,提高模型的泛化能力。
接下来,你需要使用上述定义的ResNet18_Attention模型进行训练:
``` python
model = resnet18_attention(input_shape=(32,32,3), num_classes=10)
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
lr_scheduler = keras.callbacks.ReduceLROnPlateau(monitor='val_loss', factor=0.1, patience=5, min_lr=1e-6)
early_stopping = keras.callbacks.EarlyStopping(monitor='val_loss', patience=10, restore_best_weights=True)
history = model.fit(data_generator.flow(train_images, train_labels, batch_size=128),
epochs=100,
validation_data=(test_images, test_labels),
callbacks=[lr_scheduler, early_stopping])
```
这里使用了Adam优化器,交叉熵损失函数,以及准确率作为评价指标。LR调度器和Early Stopping可以帮助优化模型的训练,避免过拟合。
最后,你可以打印出模型的准确率:
``` python
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
```
这里使用测试集对模型进行评估,输出测试集上的准确率。
完整代码如下:
相关推荐
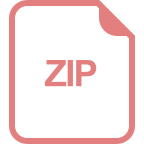
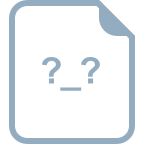
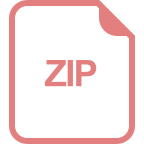














