【InputMismatchException异常和ArithmeticException异常】用Java编写一个程序,提示用户输入两个整数,然后显示它们的商。用户输入错误时提示用户重新输入,除数输入为0时提示除数不能为0。
时间: 2024-03-20 20:41:43 浏览: 21
可以使用 try-catch 语句来捕获异常,处理用户输入错误的情况。
下面是一个示例程序:
```java
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int dividend = 0;
int divisor = 0;
while (true) {
try {
System.out.print("请输入被除数:");
dividend = scanner.nextInt();
System.out.print("请输入除数:");
divisor = scanner.nextInt();
if (divisor == 0) {
throw new ArithmeticException("除数不能为0");
}
break;
} catch (InputMismatchException e) {
System.out.println("输入不是整数,请重新输入");
scanner.nextLine(); // 清除输入缓冲区
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
}
System.out.println(dividend + " / " + divisor + " = " + (dividend / divisor));
}
}
```
该程序使用了一个 while 循环,如果用户输入不正确,就会重新提示用户输入,直到输入正确为止。如果除数为0,就会抛出一个 ArithmeticException 异常,程序会捕获该异常并提示用户重新输入除数。如果输入不是整数,就会抛出一个 InputMismatchException 异常,程序会捕获该异常并提示用户重新输入。
相关推荐
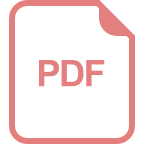
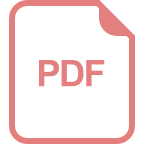











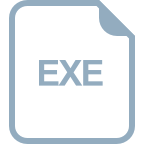
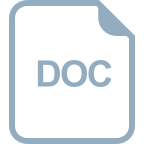
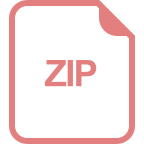
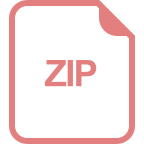